Arrays stand as vital versatile data structures that programmers heavily utilise for their programs. Arrays support data handling tasks like searching in combination with sorting and data manipulation operations at exceptional speeds when compared to alternative methods. These data structures appear frequently within C, C++ and Java programming languages when developers need to manage collections of data. The following section explains the advantages and disadvantages of array in C, C++, and Java programming languages. So, let’s get started.
What are Arrays in Java, C, and C++
Programming languages like Java, together with C and C++, employ arrays as their built-in data structure. An array maintains various values of identical type as a single grouped collection. These data structures enable simple collection and processing of information groups that share logical relationships.
To create an array in Java a programmer must start with the ‘new’ keyword and state the array data type (such as ‘int[] myList;). The array name includes parentheses containing the storage capacity to specify the element count subsequently (e.g. int[] myList = new int(10);) which creates a ten-element array. Each array maintains a fixed length which becomes immutable when allocated into memory.
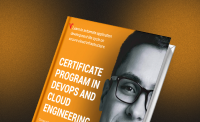
Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
Advantages of Arrays in C, C++
There are numerous advantages of array in C and C++, including:
- Allow random access
- Very easy to traverse
- Efficient memory usage
- Highly portable
- Very easy to sort
1. Random access
One of the biggest advantages of arrays in C, C++ is that they allow for random access. This means that elements can be accessed directly and in constant time, regardless of their index or position within the array. This makes it very easy to perform operations on individual elements without iterating through the entire data structure.
2. Easy to traverse
Traversal (or iteration) of arrays is also very easy. They can be traversed in order, or you can skip certain elements—all with a single loop. This makes it easy to perform various operations on the data stored within them.
3. Efficient memory usage
C and C++ allocate array data points by placing them directly after one another in a continuous memory segment. It provides efficient access to data by decreasing the time necessary for accessing any element through its contiguous storage format. The alignment of elements in one memory block enables space efficiency in data storage. The optimisation of memory usage during large dataset processing makes arrays superior to linked lists as data structures.
4. Portable
Arrays establish platform-independent data representations which enable the same data type to maintain cross-device compatibility without limitation. All C/C++ compilers support arrays which makes them suitable for any programming task requiring high complexity. Arrays have the advantage of being capable of integration with existing codebases so developers can complete new updates without needing grand-scale code modifications.
5. Easy to sort
The sorting ability of arrays becomes simple because most sorting algorithms adopt arrays as their fundamental data structure. In C++, you can harness the power of “sort” or “qsort” functions for immediate array sorting operations. The system provides programmers with an easy method to maintain and organise data according to specific requirements.
Disadvantages of Arrays in C, C++
Apart from the mentioned advantages of array, there are some disadvantages of array in C and C++. Some of the disadvantages of array are:
- Fixed size
- Lack of flexibility
- Wasted memory
1. Fixed size
Arrays are fixed, meaning that it cannot be changed once its size is determined at the time of declaration. This can be a major limitation if the size of the array needs to be flexible as per requirement.
2. Lack of flexibility
Arrays are static and cannot be dynamically re-sized. Once an array is declared, its size remains constant throughout the program’s lifetime. This can lead to wasted memory if the array size is larger than what is needed or limited space if it needs to be bigger.
3. Wasted memory
A major disadvantage of using arrays in C, C++ is memory wastage. When an array is declared, its size must be fixed and allocated at compile time. This means that all elements in the array need to be initialized even if they are not used. For instance, if you declare an array with a size of 10, but only need to use 5 elements at runtime; then 4 elements will remain unused and wasted.
Advantages of Array in Java
Here are the major advantages of array in Java:
- Easy to use
- Allow random access
- High level of flexibility
- Very high performance
1. Easy to use
Arrays are the simplest form of data structures and are easy to use. They allow us to store and access elements in a contiguous memory block.
2. Random access
Arrays allow us to access elements via their index randomly. This makes finding and retrieving data from an array much easier than with other data structures, such as linked lists or trees.
3. Flexibility
Arrays in Java offer a high level of flexibility when organizing data. Arrays can store any data, making them useful for storing and sorting large amounts of information. Also, arrays are dynamic, meaning their size can change at runtime. This allows programmers to easily add or remove elements from an array without altering the underlying code structure. As a result, developers have more freedom and control over how they use and manage their data sets.
4. Performance
The performance of array is very good as it allows random access to the elements. As the elements are continuous blocks in memory, searching an element can be done in constant time O(1) using index (i.e, direct referencing).
Also Read: One Dimensional Array in C
Disadvantages of Array in Java
The disadvantages of array in Java are as follows:
- Fixed size
- Lack of flexibility
- Overhead time & memory
1. Fixed-size
The size of an array is fixed. Once the size is declared, it cannot be changed. This may lead to wastage of memory if size allocated for array at declaration time is more than required or you might get Array Index Out Of Bounds Exception if the size allocated for an array is less than required.
2. Lack of flexibility
Arrays demonstrate limited flexibility because they come with set dimensions. If you want to add more data, you cannot do it in this array. You must create another array with larger capacity and copy old array elements into the new one.
3. Overhead
Working with arrays results in overhead expenditures of time and memory resources. Before creating an array programmers must predict a fixed amount of memory that will automatically get allocated despite the unknown size of stored data.
Scope of Arrays
Computer programming uses arrays as data structures to store homogeneous data elements sequentially at adjacent memory points. Arrays maintain substantial holdings of data which find frequent application in matrix multiplication algorithms alongside sorting and searching methods and numerous computational operations. Arrays provide quick access to specific items together with enhanced operation speeds because of their established element count.
Arrays function notably well to gather similar data entries into one group so you could utilise them to save school grades from several subjects or maintain pairs of country names and capital cities. Arrays serve advanced mathematical purposes for multiplication calculations involving matrices and linear algebraic computations.
Also Read: What is Arrays in C, C++
Challenges Faced While Using Arrays
Using arrays in programming can be quite beneficial, as they can easily store and manipulate data. However, there are some challenges associated with using arrays, such as:
1. Memory Limitations
Arrays are limited by the amount of memory allocated. As such, they may need to be reallocated or resized if the data set is larger than expected.
2. Access Time
Depending on how an array is structured, accessing elements can take longer as searching for a particular element requires looping through every element in the array individually.
3. Insertion and Deletion Times
Adding new elements to an array requires shifting existing elements, and this process can take some time, depending on the size of the array. Similarly, deleting elements from an array also requires shifting existing elements, which can increase processing time.
Conclusion
Arrays present an efficient data structure in C, C++ and Java which offers effective storage together with fast access capabilities and simple traversal methods. There are many advantages and disadvantages of array in C,C++, and Java. Arrays continue to have vital significance in data management applications because of their efficiency in handling structured information. The Certificate Program in Application Development by Hero Vired of Hero Vired provides ideal education for advanced programming proficiency. You can learn programming languages from their fundamentals to their professional level through this course
FAQs
Arrays allow for better data storage and retrieval in programs, as they are a
type of data structure that stores multiple values in one variable. This means data can be accessed quicker than if stored across multiple variables, improving program efficiency significantly.
Yes, arrays can be resized in C, C++, and Java. In C and C++, you can use the realloc() function to allocate a new memory block with a larger size dynamically. This memory is then used to store the original array plus any newly added elements. Similarly, in Java, you can use the ArrayList class, which provides methods for dynamically adding and removing elements from an array.
In C and C++, the maximum size of an array is determined by the amount of memory a program can access. The practical limit is often much lower than the theoretical limit because of memory fragmentation. In general, arrays can be at most 2 gigabytes in size. In Java, the maximum size of an array is slightly less restrictive and depends on the Java Virtual Machine (JVM). Typically, it ranges from 8 megabytes to 64 terabytes depending on the platform.
To access an element in an array, you must first determine the index of the element you are trying to access. The index is determined by counting each element from left to right starting with 0. After determining the index, use bracket notation and specify the index number inside the brackets.
A static array is a fixed-size, one-dimensional collection of elements all containing the same data type. This means that once initialized and assigned with values, its size cannot be altered. Conversely, dynamic arrays provide more flexibility as they can expand or decrease in size according to your needs during the course of program execution.
Updated on February 17, 2025