Today, data structures are an essential part of computer science. Why are they necessary? Because computers process data, you need to store and access that data in the best possible way. And that’s why data structures exist.
This beginner guide will walk you through the basics of data structures in Java. Data structures in java are a cornerstone of programming and a necessary building block if you want to understand more advanced topics. Throughout this guide, you will gain a greater understanding of what is data structures, Java data structure, advantages and disadvantages of data structures in java.
What is Java?
Java is object-oriented, meaning it uses objects as the foundation of its programming model. Objects are similar to real-world objects in that they store data and have methods (actions) that perform operations on the data stored within them.
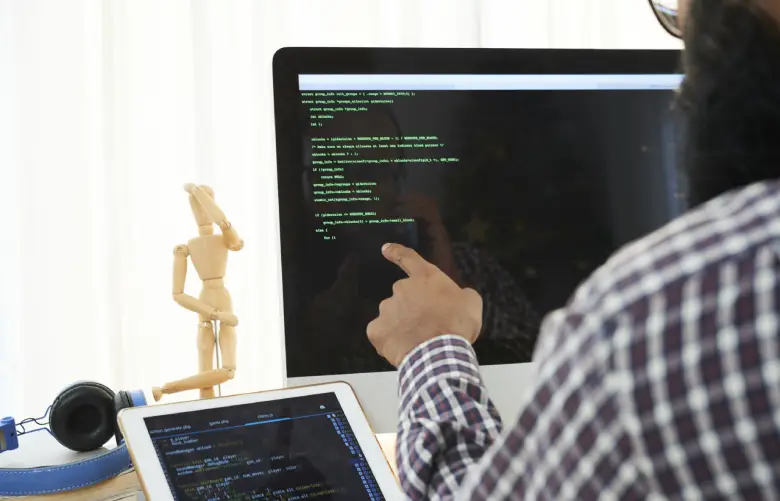
Today, Java is used by many companies and developers all over the world—including Google, Salesforce, Twitter, Amazon, Netflix, and more. Now that we have an understanding of what is Java, let’s learn more about data structures in Java and types of data structures.
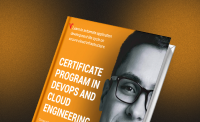
Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
Why Java is Different from other Languages?
Java is a general-purpose language that can be used for any kind of programming. It is a high-level language, which means it’s easy to read and understand by humans. It has a lot of features that make it easy to use, like the object-oriented programming model, which allows you to create objects that have their own data types, methods, and variables. Java also has an exception handling system which makes it easier to handle problems when they occur in your code.
Java has several features that make it different from other programming languages:
- Java programs are compiled rather than interpreted, which means they can be executed faster than other languages.
- Java programs use bytecode, which is the intermediate representation of a program’s instructions before it is compiled into machine code for execution on a physical processor. Bytecode is more compact than machine code, which makes it possible for Java programs to run on mobile devices or embedded systems with limited memory and processing power.
- Java uses garbage collection instead of manual memory management like many other languages do; this means developers don’t have to manually free up memory when they’re done with it because their system will do so automatically while they continue working without interruption!
What are Data Structures in Java?
Data structures in Java are the building blocks of any program. Data structures are used to organize and store data in an efficient manner. The most common data structure in java is the Array, which is a collection of objects stored in a single variable.
Data structures in java are used to store information about anything, from people to animals to cars. In this article, we will go over some of the most common data structures in Java.
Classification of Data Structure in Java
Here is the list of some of the common types of data structures in Java:
- Array
- Linked List
- Stack
- Queue
- Binary Tree
- Binary Search Tree
- Heap
- Hashing
- Graph
Data structures in java are a way to organize data in a computer so it can be used efficiently. A data structure provides information on how to store and retrieve individual elements of data.
Array
Arrays are one-dimensional java data structures consisting of consecutive memory locations that can be accessed using an index number (or subscript) as an offset address into that memory location.
Linked List
Linked lists are nonlinear data structures in java that store information in no particular order. They consist of nodes linked together in a sequence to form a chain or a circle. A linked list offers flexible storage space utilization and traversal capability because any element can be modified without affecting other elements in the list.
Stack
A stack is a container that holds its items in last-in-first-out order. This means that when an item is added to a stack, it will be added to the end of the list of items already stored in that stack. Stacks are often used when you need to run through an ordered list, or when you want to make sure that your code executes in the same order as it was written.
Queues
Queues are similar to stacks; they also hold their items in LIFO order. However, unlike stacks, queues allow items to be added at either end. This makes them useful for situations where one function may need access to resources before another function does.
Binary Tree
Binary trees are one of the most basic data structures in java. They are used to store data in a tree-like structure, where data are stored as nodes and each node is connected to only two other nodes. The first node is known as the left child and the second node is known as the right child. Binary trees can be used to store any kind of data such as numbers or characters.
Binary Search Tree
A binary search tree is a binary tree where every node has keys less than or equal to the key of its left child, and greater than or equal to the key of its right child. This means that all keys in the subtree rooted at any node are less than or equal to that node’s key and greater than or equal to that node’s key.
Heap
A heap is a special type of binary tree in which the value of each node is greater than or equal to the values of its children. Heaps are used for priority queues, where the smallest element (or more generally, an element which meets some other condition) is always removed first.
Hashing
Hashing is a method for storing and retrieving data items based on their hash value. Hash functions are used in a wide variety of applications such as text editors and dictionaries to accelerate table lookups by key. They also form the basis of data structures in java such as hash tables and hash codes.
Graph
A graph is a data structure in java that contains nodes and edges. The nodes represent the objects in the data structure, and the edges represent relationships between those objects.
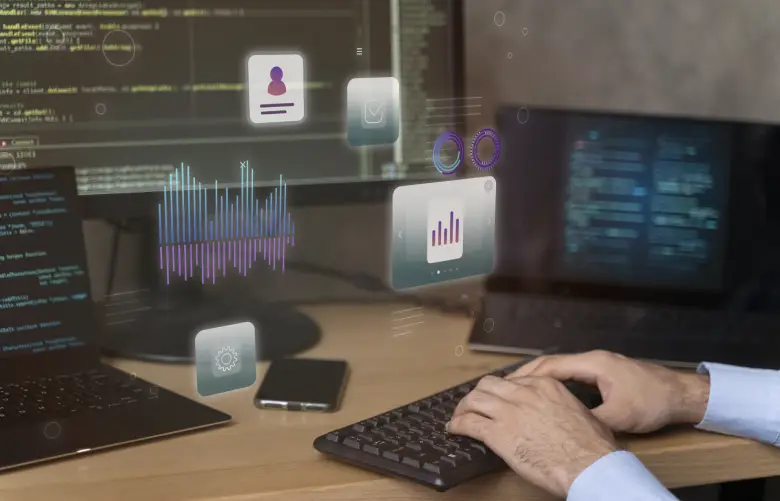
A graph is also a mathematical object that describes how vertices can be connected by edges. It is usually represented in two-dimensional space, with vertices as points and edges as lines.
The need for Data Structures in Java
Data structures in Java are very useful when you want to store and process data. Data Structures in Java help you to organize data, which means that it will be easier for you to access the information that you need.
They allow us to store data on a computer in a way that makes it easier to work with, whether that’s by making it easier for the program to find specific pieces of information or by organizing large amounts of data in an easy-to-understand manner.
Advantages of Java Data Structures
Java is a great language for beginners because it’s simple, but it also has a lot of power. If you want to develop more complex applications, you’ll need data structures in Java. Here are some reasons why data structures in Java will make your life easier:
- They’re easy to learn and use
- They’re reliable and secure
- They’re fast
- They can be used on multiple platforms
Disadvantages of Java Data Structures
Data Structures in Java are a set of data types that can be used to store and manipulate data in Java programs. They provide a way for programmers to store data in memory, and make it easy to handle memory management.
However, there are some limitations to using Java’s built-in data structures:
- Java does not support custom data types
- Java only supports primitive data types
- Java does not support user-defined classes or structs
Know why Java is the most popular language among developers.
Conclusion
This guide we have covered what is data structures in Java, the types of data structures in Java and many more. Hopefully, you now have a better understanding of the importance of data structures in Java. Along with their associated algorithms, they are vital tools to have in your arsenal when programming. Data Structures in Java will make your code clearer, more efficient, and more resistant to errors, which can save you time and energy in the long run.
HeroVired offers some brilliant courses such as Business analytics & data science course to upskill your career.
FAQs
In Java, the most commonly used data structures in Java are arrays, vectors and queues.
Recursion is a process in which a method calls itself. Recursion is used in data structures in Java to process the data in a structure, such as an array or linked list. Recursion can help you avoid using loops when you are processing a large amount of data.
The first thing you want to think about is whether or not your problem requires a tree-based structure, such as a linked list or binary tree. If so, then you'll want to use an array or linked list. If not, then you can use an array or hash table.
Updated on January 11, 2025