What are Access Modifiers in Java?
Access modifiers in Java are like security guards for class members, such as variables and methods. They determine who gets to see and interact with these members. Think of them as virtual gates controlling access to different class parts. These modifiers ensure that only the right “authorized personnel” can access specific information or functions, enhancing data privacy and code integrity. Java offers four primary access modifiers: default, private, protected, and public.
The default modifier allows access within the same package, while private restricts access to the class itself. Protected extends access to subclasses as well, fostering controlled inheritance. Public, on the other hand, opens the gates for everyone. By strategically using these access modifiers, programmers can balance sharing what’s necessary and safeguarding sensitive data. They’re essential tools for designing well-structured, secure, and manageable Java programs.
You can limit the scope of any class, method, constructor, variable, or data member using access modifiers in Java. Depending on the chosen access specifiers in Java, you will come across different benefits like security and accessibility. The Java access specifiers are often referred to as visibility modifiers.
Let’s dive deep and understand the concept of Access Modifiers in Java in detail.
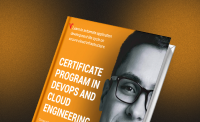
Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
How Access Modifiers in Java Work?
Access modifiers in Java are like the bouncers at a club entrance, managing who gets to enter and interact with different parts of a class. They work by setting boundaries on the visibility of class members like variables and methods. Each modifier determines which other classes or parts of the code can access these members. The public modifier acts like an open invitation, allowing any program part to freely interact with the associated class members.
The private modifier, on the other hand, keeps things exclusive, allowing only the class itself to access those members. Protected is a bit more flexible, allowing the class and its subclasses to engage with the protected members. Lastly, the default modifier keeps things within the package, granting access to other classes in the same package but preventing outsiders from meddling.
By applying these modifiers thoughtfully, programmers can create a controlled environment for their code, safeguarding sensitive information and preventing unwanted interference.
Types of Access Modifiers in Java
Now that you have understood what is access modifiers in java. Let’s look at the four types of access modifiers in Java:
1. Public Access Modifier
The public access modifiers in Java have no restriction on the instance members, methods, and classes of the particular class. When you fix classes with public access specifiers in Java, you will be able to access them in any package or class. The scope of the public Java access modifiers is extremely flexible compared to other access modifiers. This type of access modifier in java is accessible only within the class.
The public access modifier flings open the doors to class members, allowing unrestricted access from anywhere. It’s like having an open invitation to a public event. Members with public access can be accessed from any class, package, or even external applications. This is useful for creating reusable components and APIs.
Features:
- Members are accessible from anywhere in the program.
- Provides the broadest level of accessibility.
- Ideal for creating reusable components and exposing APIs.
public class PublicExample {
public int publicVar = 42;
}
2. Private Access Modifier
The private access modifiers in Java are quite restricted. Data members and class methods prefixed with only private access modifiers can only be accessed inside the class. Even other classes inside the same package cannot reach the private methods or members.
Think of the private access modifier as a private chamber accessible only by the class itself. Private members are entirely shielded from external classes, ensuring strong encapsulation and preventing unauthorized access. This is particularly useful for securing sensitive data and hiding implementation details.
Features
- Members are accessible only within the same class.
- Ensures the highest level of encapsulation.
- Protects sensitive information from unauthorized access.
Example: class PrivateExample {private int privateVar = 42;}
You came across a lot of errors because you are trying to access the private methods and members in another class.
Learn: Bagging vs. Boosting
3. Protected Access Modifier
Protected access modifiers and instance members can be used in the same package. You will be able to access them in another package using a child class. Therefore, you are required to extend the class containing protected members in another package. You won’t get direct access by creating the class object containing protected functions and members.
The protected access modifier strikes a balance between accessibility and controlled exposure. Members with protected access can be accessed within the same package and by subclasses, even in different packages. This facilitates the concept of controlled inheritance and extends visibility to specific classes.
Features
- Members are accessible within the same package and subclasses, regardless of package.
- Encourages controlled sharing among related classes and subclasses.
- Balances encapsulation with limited accessibility.
Example:
package mypackage;
class Parent {
protected int protectedVar = 42;
}
class Child extends Parent {
void someMethod() {
System.out.println(protectedVar); // Accessible in subclass
}
}
Suppose you have two packages, First and Second. The First package includes one public class and another protected prefixed method. You have two methods of accessing this method in another package:
Find out what will happen if you use these methods.
The line ob.print() created an error because you won’t be able to access the protected method beyond its own package. However, you will be able to access it by inheriting it in another class of different packages. That’s why obl.print() won’t lead to any errors.
4. Default Access Modifier
Default access modifiers in Java are given to instance members with no specified modifier. The methods and instance members with default access modifiers in Java can only be accessed in the same package. Therefore, you can call it the package private.
The default access modifier is like having a “members-only” sign on a club entrance. It’s the default setting if no other access modifier is specified. Members with default access can only be accessed within the same package. This provides a controlled environment within a package, ensuring that only related classes can interact with each other.
Features
- Members are accessible only within the same package.
- Provides a level of encapsulation within the package.
- Helpful in creating package-level functionality or restricting access to certain components.
package mypackage;
class DefaultExample {
int defaultVar = 42; // Default access modifier
}
These are the major Access Modifiers in Java. Also read: 14 Machine Learning in Healthcare
Understanding these access modifiers and their implications is crucial for designing robust and secure Java applications. By applying the right access modifier to each class member, you can ensure proper encapsulation, control access, and enhance the overall quality of your code.
Click here to check the Collections in Java.
Java Access Modifiers with Method Overriding
When it comes to method overriding in Java, access modifiers continue to play a role in controlling visibility. Method overriding allows a subclass to provide its implementation for a method defined in its superclass. However, you can’t just arbitrarily change the overridden method’s visibility.
The rule is that you can’t reduce the visibility of an overridden method in the subclass. For example, if a method in the superclass is declared as public, you can’t make it protected or private in the subclass. But you can increase the visibility. If the superclass method is protected, you can override it with a public method in the subclass.
This ensures that the contract established by the superclass is still honored by the subclass, and no unintended exposure or restriction of methods occurs during the inheritance process.
Algorithm to Use Access Modifier in Java
The different algorithms for using access modifiers in Java are as follows:
- Define a class: It revolves around making a class that will signify the object you need to manage.
- Mention access modifiers in Java: You need to specify access modifiers in Java for every instance so that it can determine the visibility of the variable.
- Define instance variables: You need to define instance variables within the class representing the data that needs to be managed.
- Use public for variables that must be accessible from anywhere: The public java access modifiers are helpful for allowing access to a variable from anywhere. It is the least limited Java access modifier with the lowest amount of encapsulation.
- Use accessor and mutator approaches for managing variable access: You should use the accessor and mutator methods to access and modify variables. It delivers a level of abstraction and makes any code more testable and maintainable.
- Identify Scope: Determine your class member’s intended level of visibility (e.g., variable, method).
- Apply Modifier: Select the appropriate access modifier (default, private, protected, public) based on your visibility requirement.
- Place Modifier: Add the chosen modifier before the member’s declaration.
- Consider Context: Consider the class’s role and interactions within your program.
- Balance Access: Use access modifiers to balance revealing enough for external use and keeping internal details secure.
By thoughtfully applying access modifiers, you ensure that your class members are accessible only to those who need them, contributing to a well-structured and secure codebase.
Quick Comparison of Types of Access Modifier in Java
Below is the quick comparison of types of access modifier in java:
Access Modifier in Java |
Same class |
Same package non-subclass |
Same package subclassDifferent package subclassDifferent package non-subclass |
Private |
Yes |
No |
No |
No |
No |
Default |
Yes |
Yes |
Yes |
No |
No |
Public |
Yes |
Yes |
Yes |
Yes |
|
Protected |
Yes |
Yes |
Yes |
Yes |
No |
Let us see which all members of Java can be assigned with the Java Access Modifiers
Below are the key members of Java can be assigned with the Java Access Modifiers:
Members of JAVA |
Private |
Default |
Protected |
Public |
Class |
No |
Yes |
No |
Yes |
Variable |
Yes |
Yes |
Yes |
Yes |
Method |
Yes |
Yes |
Yes |
Yes |
Constructor |
Yes |
Yes |
Yes |
Yes |
Interface |
No |
Yes |
No |
Yes |
Also read: Java Interview questions
Conclusion
Access modifiers in Java are primarily used for encapsulation. It allows us to control what part of a program is useful for accessing class members. The different access modifiers in Java can prevent the misuse of data. So, learn in detail about the different Java access specifiers. In this article we have understood about the java access modifiers, types of access modifiers in java and with their examples.
FAQs
Access modifiers in Java are useful for tackling the visibility of methods, fields, and constructors inside a class. Access modifiers act as gatekeepers, allowing only authorized classes or subclasses to interact with specific members. Java has four main access modifiers: default, private, protected, and public.
There are not 12 modifiers in Java; there are only four main access modifiers: default, private, protected, and public. These access modifiers can be combined with non-access modifiers like static, final, abstract, etc., to create different variations in behavior and accessibility for class members. These modifiers provide a versatile toolkit for designing classes with the desired visibility, behavior, and security level.
The different access modifiers in Java are private, public, protected, and default.
Access modifiers in Java are useful for encapsulation purposes. They also offer different types of restrictions as per requirements. It ensures that you easily protect sensitive information from direct access.
Access modifiers regulate the accessibility of class members, whereas non-access modifiers influence the behavior of these members. Access modifiers determine who can see and use class members, while non-access modifiers modify the behavior or properties of those members.
Access specifiers are crucial for maintaining data integrity, enhancing security, and promoting well-structured code. They prevent unauthorized access to sensitive data and ensure that only the intended parts of a program can interact with specific class members. Access specifiers also encourage proper encapsulation, allowing developers to control the visibility of internal details while providing well-defined interfaces for external usage.
The private and protected modifiers are not used directly for the entire class. They are meant to control the visibility of individual class members (fields, methods), rather than the class itself. The public and default (package-private) modifiers are the ones applicable to classes, allowing them to be accessed from anywhere (public) or within the same package (default).
Updated on October 8, 2024