C has been an indispensable part of computer science from the roots laid in the early 1970s. It was designed by Dennis Ritchie at Bell Labs. C was designed to be a general-purpose language capable of performing low-level operations and including high-level programming capabilities. With this unique combination, C had a huge success, and it became a touching read for a lot of modern programming languages such as C++, Java, and Python.
The historical significance of C is not what makes C important. Today, we see C used in a variety of applications, from operating systems like Windows and Linux to embedded systems in different electronics. Its influence is only too pervasive, and its relevance remains undiluted.
The Procedural Nature of C Programming
One of the discrete features of c language is its procedural nature. Procedural programming divides the problem into a set of steps (or procedures). A procedure or function does certain work. It is an intuitive way to write and even helps manage and organise complex codebases.
C uses an incremental approach to solving a problem. We observe this when we look into how functions are defined and called. A C program contains a greater number of functions, and each function does different work. Due to this modularity, the code is easier to debug, test, and maintain.
Procedural programming contrasts with object-oriented programming, which focuses on objects and data. While OOP has its advantages, the procedural approach in C provides direct control over hardware and memory. This makes it ideal for system-level programming. This control allows us to write efficient and optimised code, which is crucial for developing operating systems, compilers, and other performance-critical applications.
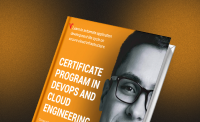

Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
High Speed and Efficiency in C Language
Speed and efficiency are the hallmarks features of c language. Several factors contribute to its performance:
- Static Typing: C is statically typed, meaning variable types are known at compile time. This allows the compiler to optimise the code effectively.
- Compiled Language: C programs are compiled into machine code, which the computer’s hardware can execute directly. This process is faster than interpreting code line by line, as is the case with languages like Python.
- Minimalistic Design: C provides only essential features, reducing overhead. Unlike modern languages with extensive libraries, C focuses on simplicity and speed.
These factors make C ideal for high-performance applications like operating systems, game development, and real-time systems. By writing efficient code, we ensure that programs run quickly and use resources effectively.
Simplicity and Ease of Understanding
C’s simplicity is one of its greatest strengths. Its syntax is straightforward, making it easy to learn and use. This simplicity does not limit power; it provides a solid foundation for understanding more complex programming concepts.
- Clean Syntax: C’s syntax is clean and minimalistic, avoiding unnecessary complexity. This clarity helps new programmers grasp the basics without confusion.
- Educational Value: C is often used as an introductory language in computer science courses because of its simplicity. Learning C gives students a strong understanding of fundamental programming principles.
- Focus on Core Logic: By stripping away extraneous features, C allows us to focus on the core logic of our programs. This focus leads to better problem-solving skills and a deeper understanding of how computers work.
Modularity and Structured Programming Approach
Modularity is among one of the most important features of c language. One is that it enables us to identify key sub-programs in large and complicated programs. These parts, known as functions, can, therefore, be written, compiled, and even tested without the need for other components to be present. This approach is also easier and less time-consuming to use in the development process.
In C, functions are the standard means of partitioning work. Every single function has its own operation and can be used in other locations of the program. This not only saves time but also space, which is translated into enhanced code readability. For instance, suppose we require a formula for performing a mathematical computation that must be computed several times; this is when one has to define a function and call it.
This modular approach is strengthened by the structured programming of C. This way, we divide codes into logical sections and make them easier to understand and maintain. It also minimises errors that might be made in writing program codes. For example, instead of typing many lines of complicated code, we split the actual code into different sections. Each section addresses a particular task, and thus, locating and correcting errors becomes less of a challenge.
Here are some benefits of modularity and structured programming in C:
- Improved Readability: Smaller, well-defined functions are easier to read and understand.
- Reusability: Functions can be reused in different programs or parts of the same program.
- Maintainability: Modular code is easier to update and maintain.
- Debugging: Isolating issues becomes simpler when code is divided into functions.
Recursion in C Programming
Recursion is a complex aspect of C that allows a function to call itself repeatedly. This technique is most appropriate in situations where the problem can be formulated as a number of related sub-problems. Recursion also enables us to write simple but efficient programs for computing substantial problems.
For instance, when computing a number’s factorial would pose a difficult problem. Using recursion, the number can be multiplied by its factorial minus one: If the number is different from 1, it would then call itself the function while passing the number and (number factorial – 1) as arguments. This approach enhances the ease of controlling the code since it is easier to understand most of the time.
Recursion is also useful in cases, for instance, in tree traversals, where every node might have child nodes that need to be processed in the same way. Indeed, by invoking the function recursively, the search across the entire tree takes very little time.
Benefits of recursion in C programming include:
- Simplicity: Reduces the complexity of code for problems that have repetitive structures.
- Readability: Makes the logic of the program clearer and easier to follow.
- Efficiency: Solves complex problems with fewer lines of code compared to iterative solutions.
Portability Across Different Systems
One of the reasons this programming language has been popular to date is that it is portable. C language programs can easily be made to work on different types of hardware platforms because C is a machine-independent language. This implies that the same C code can be run on different platforms, as it is also compiled differently on each platform.
For example, if we develop a program on Windows, we can code it for Linux with only minor modifications. Such portability makes C suitable when developing software that needs to run on different platforms.
This portability is largely afforded by the ANSI C standard. If we adhere to the ANSI C standard, our programs will be portable across different compilers and platforms. This also helps reduce the level of effort required for adapting code from one environment to another.
Benefits of C’s portability include:
- Cross-Platform Compatibility: Code can run on different systems with minimal changes.
- Efficiency: It saves time and resources when developing software for multiple platforms.
- Standardisation: Ensures consistency and reliability across different environments.
Dynamic Memory Management in C
Another notable feature of c language is dynamic memory management, by which memory can be allocated and freed during program execution. This flexibility is paramount, especially in applications where space usage is dynamic in nature and, therefore, requires efficient memory usage.
In C, the process of allocating memory dynamically is done by various functions such as malloc(), calloc(), realloc(), and free(). These functions assist in the process of assigning the needed amount of memory space and freeing it when necessary. This approach eliminates memory wastage and, at the same time, enhances the efficiency of the program in execution.
Dynamic memory management becomes very important when we require the exact amount of memory in advance. For instance, when using data structures such as a linked list or processing user inputs, dynamic memory allocation is important to utilise memory as optimally as possible.
Here are the key functions used in dynamic memory management:
- malloc(): Allocates a specified number of bytes and returns a pointer to the allocated memory.
- calloc(): Allocates memory for an array of elements, initialises them to zero, and returns a pointer to the memory.
- realloc(): Resizes the previously allocated memory block.
- free(): Deallocates the previously allocated memory, making it available for future use.
Advantages of dynamic memory management in C:
- Efficient Resource Utilisation: Allocates memory as needed, avoiding wastage.
- Flexibility: Allows programs to handle varying memory requirements.
- Performance Optimization: Improves the performance of applications by managing memory efficiently.
The Power and Utility of Pointers
Pointers are considered one of the most valued and useful features of c language. They give a direct link to memory and offer a way to process data optimally. A pointer is a variable that holds the memory address of some other variable. This means that by using pointers, we can manipulate memory and different kinds of data structures more effectively.
Pointers are required for dynamic memory allocation, arrays, linked lists, trees, and almost every data structure. They allow for the creation of intricate data structures and their organisation and use. For instance, pointers enable the creation of linked lists, which is an easy way of storing data since elements embedded in the list contain pointers to the next elements.
Another way pointers can be employed is to pass large amounts of data to functions without making a copy. This minimises the program’s overhead while enhancing its performance. Moreover, pointers are necessary for low-level operations like working with hardware or optimising basic parts of the code.
Key uses of pointers in C include:
- Dynamic Memory Allocation: Manage memory dynamically using functions like malloc() and free().
- Data Structures: Create and manipulate complex data structures like linked lists, trees, and graphs.
- Function Arguments: Pass large data sets to functions efficiently without copying them.
- Memory Manipulation: Access and modify memory directly for performance optimisation.
Benefits of using pointers in C:
- Flexibility: Provides a flexible way to manage memory and data structures.
- Efficiency: Reduces overhead by avoiding unnecessary data copying.
- Performance: Enhances performance by allowing direct memory access and manipulation.
Comprehensive Built-in Operators and Functions
C language provides a wide range of inbuilt operators and functions, which further enhances the computational power of the language to solve different programming problems effectively. These operators and functions constitute the fundamental part of any C program and help in manipulating data effectively. They are of different operators, all of which have a particular function. These operators can be classified into several categories:
- Arithmetic Operators: These operators perform basic mathematical operations.
- Example: +, -, *, /, %
- Usage: int sum = a + b;
- Relational Operators: They compare two values and return a boolean result.
- Example: ==, !=, >, <, >=, <=
- Usage: if (a > b) { … }
- Logical Operators: These operators are used to perform logical operations.
- Example: &&, ||, !
- Usage: if (a && b) { … }
- Bitwise Operators: These perform operations at the bit level.
- Example: &, |, ^, ~, <<, >>
- Usage: int result = a & b;
- Assignment Operators: They assign values to variables.
- Example: =, +=, -=, *=, /=, %=
- Usage: a += b;
- Increment and Decrement Operators: These increase or decrease the value of a variable.
- Example: ++, —
- Usage: ++a;
- Conditional Operator: A shorthand for an if-else statement.
- Example: ? :
- Usage: int max = (a > b) ? a : b;
- Comma Operator: Used to separate two expressions.
- Example: a = (b = 5, b + 2);
Standard Functions in C
C also provides a wide array of standard functions, which simplify many programming tasks. These functions are part of the C standard library and are accessible through the #include directive.
- Input and Output Functions: Functions like printf, scanf, puts, gets, and fgets help handle input and output operations.
- Example: printf(“Value: %dn”, value);
- String Handling Functions: Functions like strlen, strcpy, strcat, strcmp handle string manipulation.
- Example: strcpy(destination, source);
- Memory Management Functions: Malloc, calloc, realloc, and free manage dynamic memory.
- Example: int *ptr = (int *)malloc(10 * sizeof(int));
- Mathematical Functions: Functions like sin, cos, sqrt, and pow perform mathematical calculations.
- Example: double result = sqrt(number);
- Character Handling Functions: Functions like isalpha, isdigit, tolower, toupper deal with character manipulation.
- Example: if (isalpha(ch)) { … }
- Utility Functions: Functions such as printf, memset, memcpy, and strlen offer additional utilities.
- Example: memset(array, 0, sizeof(array));
Extending Functionality with Rich Libraries
C’s standard libraries and header files provide a rich set of functionalities that extend its capabilities. We can access these libraries by including the right headers, enhancing our program’s features and performance.
Standard Libraries in C
- h: Contains functions for input and output.
- Example: #include <stdio.h>
- h: Provides functions for memory allocation, process control, and conversions.
- Example: #include <stdlib.h>
- h: Includes functions for string handling.
- Example: #include <string.h>
- h: Provides mathematical functions.
- Example: #include <math.h>
- h: Contains functions for character handling.
- Example: #include <ctype.h>
Using Header Files
Including the necessary header files at the beginning of the program is essential. This ensures that all required functions and definitions are available for use. By including these headers, we can use their functions directly, enhancing our program’s functionality without reinventing the wheel.
Conclusion
The C programming language stands out for its powerful features and enduring relevance. Its rich set of operators and functions simplifies complex tasks, making it easier to write efficient and effective code. The extensive standard library enhances functionality and productivity, allowing us to focus on solving problems rather than reinventing solutions.
Understanding these features of c language helps us appreciate why it remains a preferred choice for many programmers. Its blend of simplicity, efficiency, and power makes it an invaluable tool for developing robust software. By mastering C, we equip ourselves with skills that are applicable across a wide range of programming domains.
Acknowledging these features assists one in understanding why C continues to be the language of choice by many programmers. It is a perfect tool for building reliable applications because of its comprehensive combination of easy learning, fast execution, and high computing performance. By mastering C, an individual extends sets of skills that are quite useful in practically any computer programming field.
FAQs
C's low-level memory access, simple syntax, and high efficiency make it ideal for system programming. It allows direct hardware manipulation and optimised performance.
C is faster due to its statically typed nature and compiler-based execution, whereas Python and Java offer more features but with additional processing overhead.
Yes, C is a general-purpose language used in various applications, including operating systems, databases, and even photo editing software.
C's simple syntax and structured approach make it an excellent introductory language, providing a strong foundation for understanding more complex programming concepts.
C allows dynamic memory allocation using functions like malloc(), calloc(), realloc(), and free(), enabling efficient memory use and management during runtime.