An array in Python is like a versatile toolbox, crafted to efficiently and precisely handle specific types of data. It’s as if you have a super-smart tool, perfect for a particular job, making things easy and accurate. Picture it as an expert in various fields, possessing a unique skill set perfectly suited for particular data scenarios.
Array in Python is a fundamental building block in the world of programming, serving as an organised data structure that plays a crucial role in efficient computation. In Python, a versatile and widely-used programming language, arrays come in various forms, each with its unique characteristics and applications. This comprehensive guide aims to delve into the different types of arrays in Python, exploring their nuances, use cases, and providing insights into how they enhance the functionality of Python programs.
Introduction to Arrays in Python
In the expansive universe of Python programming, data structures form the basis for organising and manipulating information. Among these structures, arrays hold a special place due to their ability to store collections of elements efficiently. Unlike Python lists, which can accommodate different data types, arrays in Python are designed to work with elements of the same type. This homogeneity makes them more memory-efficient and particularly suitable for numerical operations.
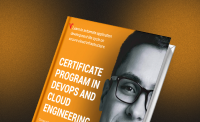
Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
Understanding the Types of Arrays
Python supports various types of arrays, each catering to specific needs and use cases. Let’s explore the major types:
One-Dimensional Arrays (1-D Arrays)
Imagine a one-dimensional array as a row, where elements are lined up one after another. This type of array is straightforward and easy to visualise. When working with a sequence of elements without complex relationships, a one-dimensional array is often the go-to choice.
Two-Dimensional Arrays (2D Arrays)
Extending the concept of a one-dimensional array, a two-dimensional array can be thought of as a matrix with rows and columns. It is essentially an array of arrays, providing a structured way to represent data that has two dimensions. This type of array is particularly useful when dealing with tables or grids of information.
Three-Dimensional Arrays (3D Arrays)
Taking complexity a step further, a three-dimensional array introduces an additional dimension, forming a cube of information. Think of it as an array of two-dimensional arrays. While less common, three-dimensional arrays find applications in scenarios where data has multiple layers of relationships.
Declaration and Initialisation of Arrays
In Python, arrays can be created using various methods. The built-in array module provides a convenient way to declare arrays with a specific type, ensuring homogeneity. During declaration, the process involves specifying the data type, array name, and size.
Common Data Types for Arrays
Different types of arrays in Python can be declared based on the data they will hold. Some common data types include:
‘i’ for signed integers.
‘f’ for floating-point numbers.
‘d’ for double-precision floating-point numbers.
‘u’ for Unicode characters.
Accessing and Modifying Elements in Arrays
Arrays in Python support operations like indexing and slicing, making it easy to access and manipulate elements.
Indexing:
In arrays, elements are accessed using indices, which represent their position in the array. Python follows zero-based indexing, where the first element is at index 0, the second at index 1, and so on.
Slicing:
Slicing allows extracting a portion of the array. It involves specifying a range of indices to obtain a subset of elements. This feature is particularly useful when working with large datasets.
Modifying Elements:
Elements in an array can be modified by assigning new values to specific indices. This capability ensures flexibility and adaptability, especially when dealing with dynamic datasets.
Use Cases and Practical Examples
- One-Dimensional Arrays: One-dimensional arrays are suitable for scenarios where data follows a linear sequence. For example, consider a one-dimensional array representing daily temperatures throughout a week. Each element corresponds to the temperature on a specific day.
- Two-Dimensional Arrays: Two-dimensional arrays shine when dealing with structured data, such as a table of student grades. The rows represent students, and the columns represent different subjects. This organisation makes it easy to perform operations on individual students or subjects.
- Three-Dimensional Arrays: In more complex scenarios, three-dimensional arrays might be used. Consider a scenario where data involves three dimensions, such as geographical coordinates (latitude, longitude, altitude). A three-dimensional array provides an efficient way to organise and process such spatial information.
Memory Efficiency and Computational Advantages
One of the key advantages of arrays in Python lies in their memory efficiency. Arrays store elements in contiguous memory locations, facilitating faster access and retrieval. This characteristic is particularly valuable in scenarios where rapid data access is crucial for performance.
Dynamic Arrays vs. Static Arrays
Arrays in Python can be categorised as dynamic or static based on their size.
- Dynamic Arrays: Dynamic arrays can adjust their size dynamically, expanding or contracting based on the data they hold. Python lists operate as dynamic arrays, making them versatile for handling varying amounts of information.
- Static Arrays: Static arrays, on the other hand, have a fixed size determined during declaration. While less flexible than dynamic arrays, static arrays offer predictability and are suitable for scenarios where the size remains constant.
Arrays in Python vs. Other Data Structures
While arrays are powerful, it’s essential to understand when to use them and when to opt for other data structures. Python provides various data structures, each serving specific purposes.
- Lists vs. Arrays: Python lists offer flexibility in accommodating different data types. However, if a more traditional array experience is desired, the array module comes into play, enforcing type consistency for situations demanding uniformity.
- NumPy Arrays: NumPy in Python, a powerful library for numerical computing in Python, introduces arrays with enhanced functionality. NumPy arrays are highly efficient for numerical operations and are widely used in scientific computing, machine learning, and data analysis.
Searching and Sorting Operations
Arrays play a pivotal role in search and sort algorithms. Two common search algorithms are linear search and binary search.
- Linear Search: Linear search involves scanning the array sequentially until the target element is found. While straightforward, its time complexity is linear, making it less efficient for large datasets.
- Binary Search: Binary search, suitable for sorted arrays, repeatedly divides the search interval, significantly reducing the number of elements to examine. This logarithmic time complexity makes it highly efficient for large datasets.
A Robust Data Structure for Efficient Programming
Python arrays, known as ‘Array in Python,’ provide efficiency and adaptability, serving as indispensable tools for streamlined data handling. As Python evolves, specialised libraries like NumPy enhance array capabilities, offering versatile solutions for diverse programming challenges. Understanding array fundamentals in Python is crucial for both beginners and experienced programmers seeking optimal performance.
Hero Vired’s Integrated Program in Data Science, Artificial Intelligence, and Machine Learning emerges as a strategic choice for those seeking a holistic and industry-centric education. With an extensive curriculum, live sessions by industry experts, and recognition by Analytics India Magazine, the program ensures a comprehensive learning experience.
The program’s standout feature lies in the thorough coverage of Python programming, a foundational skill crucial for success in these domains. By embracing this program, individuals gain theoretical knowledge, practical insights, industry connections, and career development opportunities. Elevate your expertise; embark on a transformative journey with Hero Vired. Enroll today for a future in data-driven innovation!
FAQs
In Python, three primary array types exist, each serving distinct purposes. The single-dimensional array, also known as a 1-D array, resembles a linear sequence of elements. Moving beyond this, the two-dimensional array (2D) adopts a matrix-like structure, facilitating organised data representation with rows and columns.
The three-dimensional array introduces an additional dimension, forming a cube of information and proving valuable in scenarios with intricate, multi-layered data relationships. This array of versatility empowers programmers to choose the most suitable structure for their specific data handling needs.
In Python, arrays are commonly referred to as NumPy arrays. NumPy, a powerful numerical computing library, enhances the functionality of arrays, making them highly efficient for complex mathematical operations, scientific computing, and data analysis.
To determine the object's type, utilise the type() function. Once identified as a NumPy ndarray, to ascertain the data type within the array, employ the attribute '.dtype'.
For lists doubling as arrays in Python, an empty list is formed with empty_list = []. Using the 'array' module for a stricter array definition, an empty integer array is established as follows: import array as arr; a = arr.array('i').
Within Python, lists serve as dynamic data structures, accommodating various data types. In contrast, arrays, specifically when utilised through the 'array' module, ensure a consistent data type across all elements.
This uniformity is a defining feature, offering advantages in scenarios where strict data type conformity is essential, enhancing efficiency in handling homogenous datasets. The 'array' module's emphasis on type consistency distinguishes it as a specialised tool within Python's array landscape.