Structure and Union are both user-defined data types in C that can group logically related data members of different data types together. A structure is more versatile and offers procedural functionalities, whereas a union is memory-efficient and suitable for handling unknown data types. Let’s do a deep dive and understand the concepts of structure and union in detail.
What is a Structure in C?
A user-defined data type that can group logically related data members of different data types under a single name is known as a structure. It basically represents a record. It is versatile and offers procedural functionalities, like initialising and loading multiple components simultaneously.
The total size of the structure is equal to the sum of the sizes of every data member. It can initialise multiple members concurrently, as all elements in the structure are stored in contiguous memory locations. It is defined using the struct keyword.
Syntax:
struct structure_name{
type element 1;
type element 2;
…..
type element n;
};
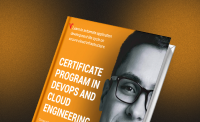

Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
Example of Structure in C
Below is an example of using structure in C language.
// Example of Structure in C
#include <stdio.h>
// define structure
struct student {
char student_name[50];
int roll_number;
int student_age;
} stdt;
// main function
int main() {
printf(“Student information:n”);
printf(“Enter student name: “);
fgets(stdt.student_name, sizeof(stdt.student_name), stdin);
// get student details
printf(“Enter student roll number: “);
// access elements using dot operator
scanf(“%d”, & stdt. roll_number);
printf(“Enter student age: “);
scanf(“%d”, & stdt.student_age);
printf(“Thanks for the information: n”);
// print the student information
printf(“Student name: “);
printf(“%s”, stdt.student_name);
printf(“Student unique id: %dn”, stdt.roll_number);
printf(“Student age: %dn”, stdt.student_age);
return 0;
}
Output:
Student information:
Enter student name: Rohan
Enter student roll number: 30
Enter student age: 14
Thanks for the information:
Student name: Rohan
Student unique id: 30
Student age: 14
In the above example, a structure called student is created, which has three data members: student_name, roll_number, and student_age. A variable stdt is created to access student information.
Also read: Types of Operators in C
Advantages of Structure in C
Below are some benefits of using structure:
- It can contain multiple variables of the same data type within the same memory location.
- It is useful when we want to collect data of similar data types and parameters.
- It is reliable, stable, and easy to maintain, as the entire record can be represented under a single name.
- It allows us to pass an entire set of records to any function by using a single name.
- We can use arrays of structures to efficiently store more records with similar data types.
Disadvantages of Structure in C
Below are some drawbacks of using structure:
- It is not recommended to use structure in complex projects, as it becomes hard to manage. Hence, it poses a problem for scalability.
- It is slower and requires dedicated memory for storing the entire data.
- Any change in data structure requires changes at multiple other places as well. This makes the process more time-consuming and difficult to track.
What is a Union in C?
A union is a custom data type that stores different data types in the same memory location. The total size of the union is equal to the size of its largest data member. In a union, only one member can be accessed at a time. Unlike structures, a union shares memory among its members, so only one element is active at a given time.
It is efficient and requires less memory when working with a large number of members. It is defined using the union keyword.
Syntax:
union union_name {
type element 1;
type element 2;
…..
type element n;
};
Example of Union in C
Below is an example of using union in C language.
// Example of Union in C
#include <stdio.h>
// define union
union employee {
char emp_name;
int emp_id;
float emp_salary;
};
// main function
int main() {
union employee emp;
// initialise data members
emp.emp_name = ‘E’;
emp.emp_id = 13243;
emp.emp_salary = 78932.45;
// print the employee information
printf(“Employee name: %cn”, emp.emp_name);
printf(“Employee id: %dn”, emp.emp_id);
printf(“Employee salary: %.2fn”, emp.emp_salary);
return 0;
}
Output:
Employee name: :
Employee id: 11293021
Employee salary: 78932.45
In the above example, the value of employee name and id gets corrupted and only employee salary is printed as expected. It happens because memory location is shared among all the members, therefore, only one data member value is currently stored.
Also read: Increment and Decrement Operators in C
Advantages of Union in C
Below are some benefits of using a union:
- It is very efficient and requires less memory.
- In a union, we can only assess the last variable directly.
- It is especially used when we want to use the same memory location for two or more data members.
- The allocated space of a union is equal to the maximum size of the data member.
Disadvantages of Union in C
Below are some drawbacks of using a union:
- A union can cause massive errors in functions due to its nature of combining every single member.
- It can use only one member at a given time.
- It assigns a single memory space to all its members, which increases complexity and causes errors when working with multiple data types.
Similarities between Structure and Union in C
Despite having many differences, they share some similarities, as listed below.
- Custom Data Types: Both structures and unions are custom data types that group different variables of multiple data types under a single name.
- Member Access: The individual members of both user-defined data types can be accessed using the dot “.” operator.
- Initialisation: Both structures and unions can be initialised at the time of declaration, just like any other variable in C.
- Memory Allocation: Both allocate memory for their members. In structure, the size is the sum of the sizes of its members, while in union, the size is calculated by the size of its largest variables.
- Assignment Operator: Both of them support assignment “=” and “sizeof” operators. For assignment, the two structures or unions must have the same number of data members and their types.
- Array Declaration: We can declare arrays of both structures and unions.
- Nested Definitions: The structures can contain unions and vice versa. It allows the creation of complex data structures.
- Functional Parameter: Both of them can be passed to functions as parameters and can be returned from functions too.
Comparison between Structure and Union in C
Here is the tabular comparison between the structure and union:
Structure |
Union |
It is defined using the struct keyword. |
It is defined using the union keyword. |
In structure, every member is assigned a unique memory location. |
In a union, all data members share a common memory location. |
The total size of the structure is equal to the sum of the sizes of every data member. |
The total size of the union is equal to the size of its largest data member. |
If the value of one data member changes, it will not affect other data members in the structure. |
If the value of one data member changes, it will affect the other data members in the union. |
It enables the initialization of several members at a time. |
It enables the initialization of only the first data member. |
It is used for storing multiple data types. |
It is used for storing any one data type. |
In structure, any member can be accessed simultaneously. |
In a union, only one member can be accessed at a time. |
Structure supports flexible arrays. |
Union doesn’t support flexible arrays. |
It is more versatile and offers procedural functionality. |
It is less versatile. |
Example:
struct structure_name{element 1;
element 2;
…..
element n;
};
struct employee{
int emp_id;
float emp_salary;
}
Example:
union union_name {element 1;
element 2;
…..
element n;
};
union student{
int studet_id;
char student_name[30];
}
Conclusion
This concludes our discussion on the difference between structure and union in C. We discussed structures and unions in detail, with their benefits and drawbacks. We saw different ways to initialise structures and unions with examples and, in the end, a tabular comparison between them to get a better understanding of the topic.
FAQs
Structures and unions are the user-defined data types that combine objects of different types and sizes together as a single unit. They basically represent a record.
Union is used to store multiple variables of different data types in defined memory locations. It is specifically used when data types are unknown.
Generally, there are two types of unions in C: tagged and untagged unions. The tagged union is capable of handling multiple variables but of fixed types, whereas the untagged union can handle multiple variables of any data type.
Structure is more reliable and consistent when working with multiple data types. However, unions are more efficient and require less memory when working with a large number of members.