Understanding the difference between compilers and interpreters in software development is crucial. A compiler can be regarded as a planner who reads entire programs, translates them to machine language, and runs them as a single program. This ensures efficiency, but execution takes time.
However, an interpreter is a live translator who interprets code line by line as the program runs. It is more flexible and responsive but slightly slower to execute.
Your development needs to determine the tool you choose. This article helps you determine which compilers and interpreters are better for your project. Understanding this is important for all software developers because it helps them decide which tools to use.
What is a Compiler?
A compiler is a program that converts high-level source code written in C, C++, or Java into machine code or binary code before execution. It transforms the codes in the programming language and finally translates them into machine code (0s and 1s) so that computers can understand them.
- An assembler is more intelligent than a compiler because a compiler checks all kinds of limits, ranges, errors, etc.
- Its program run time is longer, and the memory occupied becomes larger. The speed is slow because the compiler can not access lines of executable code directly; instead, the whole program is processed and translated into machine code.
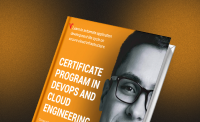
Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
Role of a Compiler
The compiler is important in programming as it acts as an interface between human-readable and machine-executable codes. A compiler converts the high-level language into an intermediate assembly language, and an assembler converts the compiler’s intermediate assembly language into machine code.
Its functions include:
- Code Translation: Converting high-level language source code such as C, C++, and Java into binary format or machine code.
- Error detection: Analyzes all source code regarding syntax or semantic errors before producing the executable.
- Translation Optimisation: The translated code should be efficient enough so that the program runs better.
- Executable File Creation: Reads the whole program as an executable single file that you can run without further translation.
Also Read: Highest Paying Programming Languages to Learn in 2025
How Does a Compiler Work?
Compilers Steps for Programming:
- Creation of a Program.
- The compiler, however, analyses language and flags any wrong statements.
- The Compiler converts the source code into Machine Code if no faults occur.
- Linking various code files together to run as a program.
- Finally, it runs the program.
Types of Compilers
1. Single-Pass Compiler
Processes the entire code in a single scan.
Faster but less efficient.
Example: Early versions of Pascal.
2. Multi-Pass Compiler
Goes through the code multiple times, applying optimisations at each step.
It is slower but produces better performance.
Example: Modern C and C++ compilers.
3. Source-to-Source Compiler
Converts code from one programming language to another.
Example: TypeScript compiler.
4. Cross Compiler
Creates programs for different systems.
Example: A Windows computer compiling code for a mobile device.
5. Native Compiler
Produces machine code specific to the system it runs on.
Example: GCC or GNU Compiler Collection for C and C++.
6. Just-In-Time Compiler
Compiles small parts of a program at runtime instead of compiling the whole code before execution.
Example: Java Virtual Machine uses JIT for Java.
7. Ahead-of-Time Compiler
Converts the whole program into machine code before execution, reducing startup time.
Example: Android’s ART or Android Runtime.
8. Optimising Compiler
Improves code efficiency by removing unnecessary operations.
Example: LLVM Compiler.
9. Bytecode Compiler
It transforms high-level code into an intermediate form (bytecode) to run it on a virtual machine.
Example: Java-JVM and Python-PVM.
10. Incremental Compiler
Only recompiles the changed parts of the code, saving time.
Example: Used in IDEs like Eclipse.
11. Bootstrap Compiler
A compiler that is written in the same language it compiles.
Example: Early versions of C compilers.
12. Decompiler
Reverses compiled code back to readable source code.
Example: Java Decompiler.
13. Language Rewriter
It converts the code from one style to another to make the same code more readable.
Example: Code formatters.
14. Assemblers
Converts assembly language into machine code.
Example: NASM or Netwide Assembler.
What is an Interpreter?
An interpreter translates and executes code line by line instead of compiling it simultaneously. This makes the debugging process easier but slows down execution.
Role of an Interpreter
Programming with an interpreter is necessary for running lines of code simultaneously, where the code can be interacted with in real time. Its key roles include:
- Line-by-Line Execution: It immediately translates and runs each line of code, thus perfect for debugging and interactive programming.
- Error Detection: Functions to identify errors while running the program so that the developers can fix the error while the entire program is going so that it does not halt.
- No Executable File: Does away with creating an executable file, which quickly tests small code changes.
- Supports dynamic programming: The developers can modify the code in execution.
Also Read: Top 10 Backend Languages to Know in 2025
How Does an Interpreter Work?
Interpreter’s Steps for Programming:
- Creation of a Program.
- The interpreter does not need to link files or Generate machine code.
- Execution of source statements one by one.
Types of Interpreters
1. Sequential Interpreter
Executes each line in the order in which it appears.
Example: Python interpreter.
2. Interactive Interpreter
Allows users to enter commands one by one and see the output immediately.
Example: Python shell, JavaScript console.
3. Batch Interpreter
Executes a script or a set of commands all at once.
Example: Bash shell.
4. Bytecode Interpreter
Converts code into bytecode before executing it.
Example: Python Virtual Machine-PVM, Java Virtual Machine-JVM.
5. Just-In-Time or JIT Interpreter
Combines interpretation and compilation for better performance.
Example: Java JIT compiler.
6. Tree-Walk Interpreter
Converts code into a tree structure and executes it step by step.
Example: JavaScript’s V8 engine.
7. Source-to-Source Interpreter
Converts code from one language to another before running it.
Example: Some SQL interpreters.
8. Hardware Interpreter
Uses hardware instructions to speed up execution.
Example: Graphics processing units.
9. Emulator or Virtual Machine Interpreter
Allows running programs designed for different systems.
Example: Android Emulator, QEMU.
10. Dynamic Translator
Translates code while it runs, adapting to different systems.
Example: JavaScript engines in web browsers.
11. Domain-Specific Interpreter
Designed for specific applications.
Example: MATLAB interpreter.
12. Concurrent Interpreter
Runs multiple parts of a program at the same time.
Example: Python’s multiprocessing module.
13. Threaded Code Interpreter
Uses pre-compiled code snippets for efficiency.
Example: Used in real-time operating systems.
14. Abstract Syntax Tree or AST Interpreter
Converts code into a tree representation before executing.
Example: Some JavaScript engines.
Also Read: Top 10 Front End Languages – A Beginner’s Guide
Compiler vs Interpreter: Advantages and Disadvantages
Advantages of Compilers:
- When considered in terms of speed and less time, the compiler runs faster than the interpreter.
- It plays a role in securing the application.
- Compilers also provide debugging tools that conveniently help develop software and fix errors.
Disadvantages of Compilers
- However, Compilers run faster than interpreters. However, working with bulk codes might take longer than expected.
- Compilers can catch some semantics errors and syntax errors only.
Advantages of Interpreter
- High-level programming languages converted into machine code by interpreters are easier to debug.
- It reduces the risk of memory error as it helps in memory management automatically.
- When looking into flexibility. Compiled language is less flexible than interpreted language.
Disadvantages of Interpreter
- The interpreter does not allow you to translate language faster.
- The interpreter can run only the corresponding Interpreted program.
Similarities between Compiler and Interpreter
- The Compiler and the Interpreter should translate the source code into machine-readable form.
- Syntax checking and generating an error message are done by Compile and Interpret.
- The compiler or an interpreter can execute a program written in a high-level programming language.
- Software development is a combination job of Compiler and Interpreter.
- The Compiler and Interpreter process the source code, and the output will be generated.
- To begin translation, the source code file has to be provided to the compiler and the interpreter.
Difference between Interpreter and Compiler
The following table compares the differences between an interpreter and a compiler based on their working, execution, efficiency, and other key aspects.
Feature |
Compiler |
Interpreter |
Execution Process |
Translates the entire program into machine code before execution, which allows it to run independently. |
Translates and executes code line by line, meaning execution stops at the first error encountered. |
Speed |
Generally, it is faster because the entire code is compiled before execution. |
It is slower because it processes each line individually during runtime. |
Error Handling |
Detects all errors after compiling the entire code, making debugging difficult. |
Identifies and reports errors one at a time, making debugging easier but slowing execution. |
Storage |
Produces an independent executable file, allowing programs to run without requiring the source code. |
It does not generate a separate executable; the source code is needed every time the program runs. |
Optimisation |
Optimises the entire code before execution, which results in better performance. |
It does not perform extensive optimisation as execution happens incrementally. |
Dependency |
Once compiled, the source code is no longer required for execution, making distribution easier. |
It always requires the source code since it translates on the fly during execution. |
Usage |
It is often used where high performance is required for system software and large-scale applications. |
It is highly preferred for scripting, debugging, and real-time code execution, such as web development or automation. |
Examples |
Compilers convert source code into machine language before execution in C, C++, and Java. |
These are the languages of dynamic execution that use interpreters like Python, JavaScript, and Ruby. |
Conclusion
The software developers form the backbone of the programming languages. To excel in the digital world, understanding the nuances between a compiler and an interpreter is fundamental. If you wish to be part of this soaring world as a developer, you must seize the opportunity by enrolling in the Certificate Program in Application Development. This certificate program gives learners all the skills and knowledge required to thrive in software development, allowing them to contribute heavily to the constantly and unstoppable evolving IT industry.
FAQs
The choice between compiler and interpreter depends totally on the work the user needs to perform. The interpreter is best for debugging, but it translates the language slowly. On the other hand, resolving errors becomes tough with the compiler.
The compiler converts the whole programming language into machine-readable codes simultaneously while the interpreter transforms and executes the process one by one, making the whole process slower.
Python is an interpreted language that transforms the
source code of a Python program into bytecode, which is subsequently executed by the Python virtual machine. In contrast to prominent compiled languages like C and C++, Python's code does not require the building and linking processes associated with these languages.
An Interpreter executes instructions written in a programming or scripting language without first translating them into object or machine code. Interpreted languages such as Perl, Python, and Matlab exemplify this approach. Below are several intriguing insights into interpreters and compilers.
Although a compiler is similar to an interpreter, it excels in speed by translating the entire file in one go. In contrast, an interpreter processes the source program line by line, resulting in a slower operation. TurboC++ and Keil are notable examples of widely employed compilers.
Execution of the complete program is made faster at the expense of compilation, and the program must be compiled before the execution. The interpreter translates and executes code line by line, which is quicker but harder to debug. When we say compilers will generate independent executable files, interpreters will require source code every time the program runs. The interpreters are Python and JavaScript, whereas the compiled languages are C and C++.
Updated on February 11, 2025