Object and class are the basic concepts of object-oriented programming that represent real-world concepts and entities. A class is a template that defines the properties and behaviour of an object, whereas an object is defined as an instance of a class. Let’s do a deep dive and understand the concepts of class and object in detail.
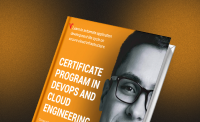
Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
What are Java Classes?
A class is a blueprint that defines properties and behaviour for object creation. It is a logical entity defined as a group of similar objects. It is declared using the class keyword and can be declared only once. No memory is allocated when a class is created.
Syntax:
<access_modifier> class <class_name>
{
data member;
method;
constructor;
nested class;
interface;
}
Example:
// Example of Class
public class Car {
// instance variable
String car_name;
// instance variable
String car_color;
// instance variable
int car_speed;
// Constructor
Car (String name, String color, int speed){
car_name = name;
car_color = color;
car_speed = speed;
}
// Member method to initialize data members
void initialize_object(String name, String color, int speed){
car_name = name;
car_color = color;
car_speed = speed;
}
}
What are the components of a Java Class?
In Java, class declaration generally includes the following components:
- Modifiers: A class can have public or default access.
- Class Keyword: A keyword that is used to create a class.
- Class Name: The name given to the class in camel case format.
- Member Variable: The variables defined within the class body are known as member variables. It can be of two types: class variables and instance variables.
- Member Functions: The methods defined within the class body are member functions.
What are the different types of Classes in Java?
In Java, classes can be categorized into the following types:
- Concrete Class: A regular class in which all methods of an abstract class are implemented is a concrete class. Its object can be created directly.
- Abstract Class: A class declared with an abstract keyword is known as an abstract class. It can’t be instantiated, but it can be a subclass.
- Static Class: A class declared with a static keyword is known as a static class. It can’t be instantiated. Its members can be accessed using the class name itself.
- Final Class: A class declared with the final keyword is known as the final class. These classes are immutable and can’t be extended.
- Singleton Class: A class having one object at a time is a singleton class. It is generally used to control access when dealing with database connections.
- POJO Class: POJO stands for Plain Old Java Object. A POJO class contains private variables, getters, setters, and constructors.
- Inner Class: A class defined within a class is known as an inner or nested class.
What are the uses of Class in Java?
Below are the major uses of class:
- It helps to create user-defined objects.
- It can hold both data variables and member functions.
- It can be used to inherit the properties of another class.
- It is used to take advantage of constructors.
- It is generally used in complex applications with large amounts of data.
- It provides a technique to organize information about data.
What are Java Objects?
A Java object is defined as the instance of a class. It is a basic unit of object-oriented programming. It is a real-world entity that includes attributes and behaviours associated with a certain class. It is a runtime unit. A class can have any number of objects that interact by invoking methods.
How to Declare an Object in Java?
In Java, declaring an object corresponds to making an instance of a class. All instances share the same attributes and behaviours of a class, but their values will be unique for each object.
Example:
ClsName objName = new ClsName();
ClsName: It represents the name of the class.
objName: It represents the name of a variable that holds an object.
new ClsName(): It creates a new instance of a class and assigns it to objName.
How to Initialize an Object in Java?
Initialization is the process of assigning values to the data members of a class. In Java, there are three ways to initialize an object.
Initialize an Object by using a Reference
This initialization is simply done by using an assignment operator. The reference of an object is used to store values.
Example:
// Example of object initialization using a reference
// Declare a Car class with three member variable
public class Car {
String car_name;
String car_color;
int car_speed;
}
class Main{
public static void main(String args[]){
// Create an object of Car class using new operator
Car car_object = new Car();
//Initialize the class members using reference
car_object.car_name = “BMW”;
car_object.car_color = “White”;
car_object.car_speed = 120;
// Display member variable of the class.
System.out.println(“Car Name:” + car_object.car_name);
System.out.println(“Car Color:” + car_object.car_color);
System.out.println(“Car Speed:” + car_object.car_speed);
}
}
Output:
Car Name:BMW
Car Color:White
Car Speed:120
In the above example, we initialize an object using the assignment operator.
Initialize an Object by using a Method
This initialization is simply done by invoking a member method of the class.
Example:
// Example of object initialization using a method
// Declare a Car class with three member variable
public class Car {
String car_name;
String car_color;
int car_speed;
// Member method to initialize data members
void initialize_object(String name, String color, int speed){
car_name = name;
car_color = color;
car_speed = speed;
}
}
class Main{
public static void main(String args[]){
// Create an object of Car class using new operator
Car car_object = new Car();
//Initialize the class members using member function
car_object.initialize_object(“Mercedes”, “Black”, 150);
// Display member variable of the class.
System.out.println(“Car Name:” + car_object.car_name);
System.out.println(“Car Color:” + car_object.car_color);
System.out.println(“Car Speed:” + car_object.car_speed);
}
}
Output:
Car Name:Mercedes
Car Color:Black
Car Speed:150
In the above example, we initialize an object using the member function of a class.
Initialize an Object by using a Constructor
This initialization is simply done by invoking a constructor of a class.
Example:
// Example of object initialization using a constructor
// Declare a Car class with three member variable
public class Car {
String car_name;
String car_color;
int car_speed;
// Constructor to initialize object
Car (String name, String color, int speed){
car_name = name;
car_color = color;
car_speed = speed;
}
}
class Main{
public static void main(String args[]){
// Create an object of Car class using new operator
// and initialize it with constructor
Car car_object = new Car(“Rolls Royce”, “Red”, 180);
// Display member variable of the class.
System.out.println(“Car Name:” + car_object.car_name);
System.out.println(“Car Color:” + car_object.car_color);
System.out.println(“Car Speed:” + car_object.car_speed);
}
}
Output:
Car Name:Rolls Royce
Car Color:Red
Car Speed:180
In the above example, we initialize an object using the constructor of a class.
How to Create an Object in Java?
In Java, there are four ways to create an object.
Using a new Keyword:
The most common method to create an object is to use a new keyword followed by the constructor of the class.
Example: ClsName objName = new ClsName();
In the above example, an object of “ClsName” is created and assigned to “objName” .
Using the Class.forName() Method:
Java provides a class known as “Class” that keeps all the information about the classes and objects in the system. The forName() method of the “Class” class can be used to create an object; we need to pass the class name as a parameter, and a new instance of the class is returned.
Example: ClsName objName = (ClsName) Class.forName(“ClsName”).newInstance();
The above example will return a new object “objName” of class “ClsName”.
Using the clone() Method:
In Java, the clone() method is used to create a new object by cloning the existing object.
Example: ClsName objName1 = new ClsName();
clsName objName2 = objName1.clone();
In the above example, clone() method creates a new instance, “objName2”, which is a copy of “objName1”.
Using Object Deserialization:
Deserialization is a technique for reading an object from a saved file. An object can be created by deserializing it from a file system.
Example: objectInputStream objInputStream = new ObjectInputStream(inputStream);
ClsName objName = (ClsName) objInputStream.readObject();
In the above example, “objInputStream” reads serialized data from “inputStream,” which is later deserialized and assigned to “objName.”
What are the uses of Objects in Java?
Below are the major uses of objects:
- Objects are used to manipulate data.
- It can access the piece of memory using an object reference variable.
- It represents a real-world problem for which we find a solution.
- It allows data members and member functions to perform desired tasks.
- It helps to find the type of message accepted and the type of response returned.
Comparison between Class and Object in Java
Here is the tabular comparison between the class and the object:
Class |
Object |
A class is a blueprint that defines properties and behaviour for object creation. |
An object is defined as an instance of a class. |
It is a logical entity. |
It is a physical entity. |
No memory is allocated when a class is created. |
Memory is allocated when an object is created. |
A class can be declared only one time. |
There can be more than one object using a class. |
It is declared using a class keyword. |
It can be created using a new keyword, forName(), and clone() methods. |
It can’t be manipulated. |
It can be easily manipulated. |
It is a group of similar objects. |
It is a real-world entity such as a book, car, etc. |
It generates objects. |
It provides life to the class. |
Conclusion
This concludes our discussion on the difference between objects and classes in Java. We discussed objects and classes in detail, their types, and their usage. We saw different components of a class, different ways to initialise and create an object with examples, and, in the end, a tabular comparison between them to get a better understanding of the topic.
FAQs
In Java, an object is defined as the instance of a class that represents a real-world entity, and a class is defined as the blueprint that defines the structure and behaviour of objects.
In Java, we can create an object using constructors, object initialization blocks, cloning, and deserialization.
In Java, we can create an instance of a class using the “new ” keyword followed by the class constructor.
Example: className obj = new className();
A class acts as a blueprint that contains properties and behaviours for creating objects.
An object that is created without being assigned to any variable is known as an anonymous object. It is generally used for a single operation.