Merge sort is the efficient and stable sorting algorithm in C to sort the elements of an array. It uses the divide and conquer method to sort the array elements. Merge Sort operates in the following steps:
- Divide:
The array is recursively divided into two sub-arrays until each half is left only with a single element.
- Conquer:
Next, each sub-array is sorted individually. Here, the base case of the recursion is reached when each sub-array has one element, meaning it is already sorted.
- Combine:
The sorted sub-arrays or halves are then merged back together to form a larger sorted array.
Let’s see the working of the merge sort algorithm in detail and with an example:
Consider an array of elements: [53, 24, 35, 76, 12, 5, 3, 6, 23]
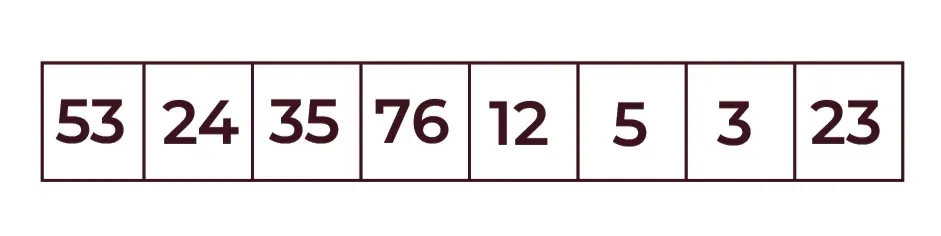
Step 1: Divide the array into sub-arrays (two halves):
firstHalf: [53, 24, 35, 76], secondHalf: [12, 5, 3, 6, 23]
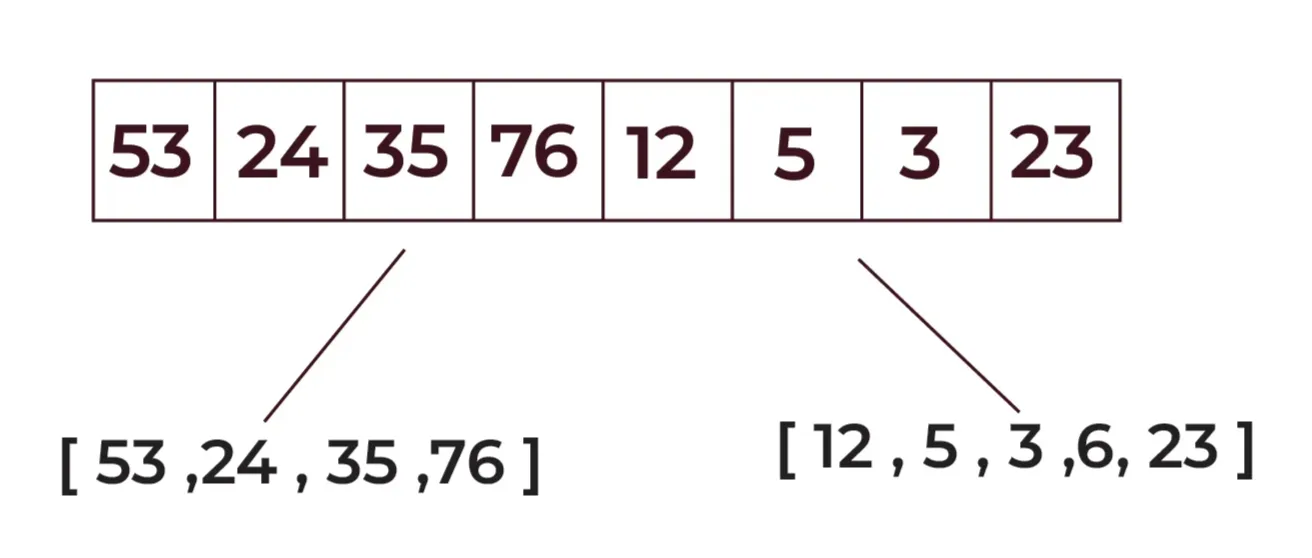
Step 2: Now recursively divide the sub-arrays:
firstHalf: [53, 24, 35, 76] becomes [53, 24] and [35, 76]
secondHalf: [12, 5, 3, 6, 23] becomes [12, 5] and [3, 6, 23]
Step 3: Continue dividing (splitting) the arrays until each sub-array is left only with a single element:
[53, 24] becomes [53] and [24]
[35, 76] becomes [35] and [76]
[12, 5] becomes [12] and [5]
[3, 6, 23] becomes [3] and [6, 23]
[6, 23] becomes [6] and [23]
Now, each array element is split till all of them are left individually in the array. It is now ready to merge into one array again after doing sorting.
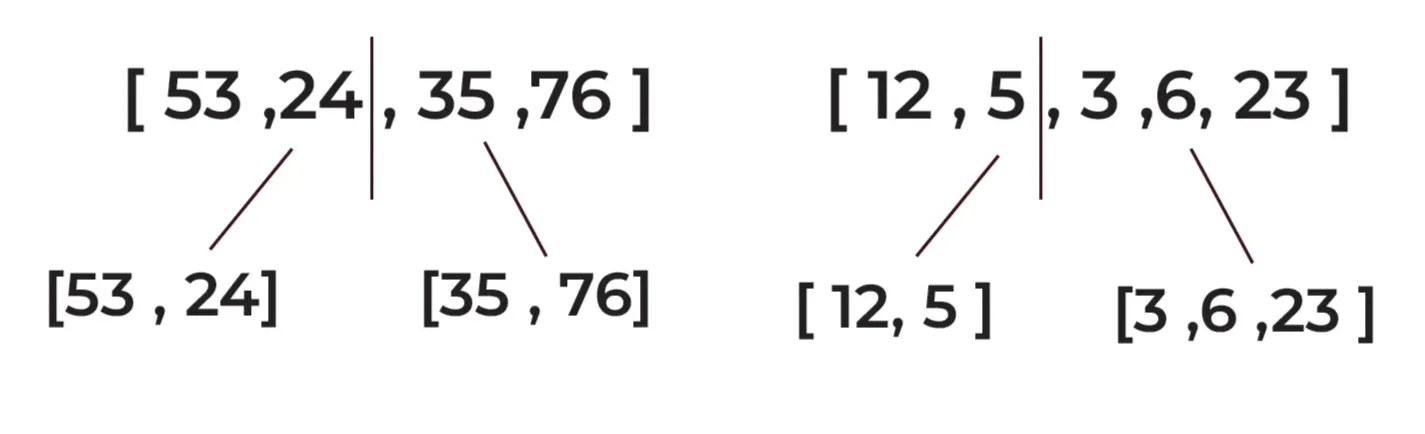
Step 4: Merge the sorted sub-arrays:
Merge [53] and [24] to get [24, 53]
Merge [35] and [76] to get [35, 76]
Merge [12] and [5] to get [5, 12]
Merge [6] and [23] to get [6, 23]
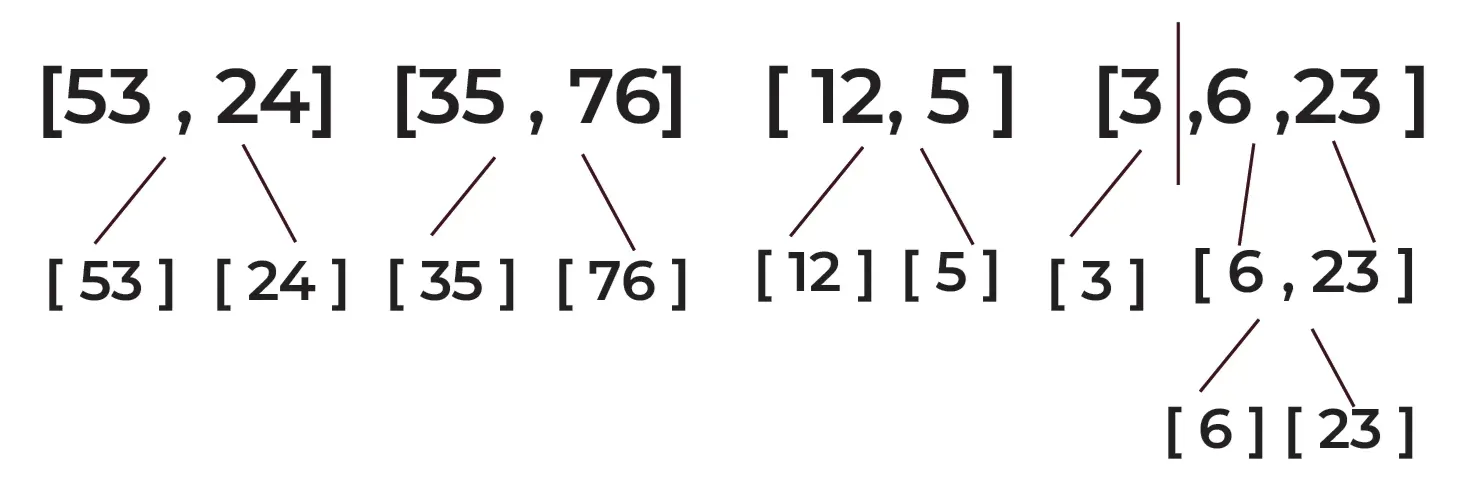
Step 5: Merge the final two sub-arrays to get the sorted array:
Merge [24, 53] and [35, 76]
Compare 24 and 35: Take 24
Compare 53 and 35: Take 35
Compare 53 and 76: Take 53
Remaining elements: 76, Output: [24, 35, 53, 76]
Merge [5, 12] and [3, 6, 23]
Compare 5 and 3: Take 3
Compare 5 and 6: Take 5
Compare 12 and 6: Take 6
Compare 12 and 23: Take 12
Remaining elements: 23, Output: [3, 5, 6, 12, 23]
Merge [24, 35, 53, 76] and [3, 5, 6, 12, 23]
Compare 24 and 3: Take 3
Compare 24 and 5: Take 5
Compare 24 and 6: Take 6
Compare 24 and 12: Take 12
Compare 24 and 23: Take 23
Remaining elements: 24, 35, 53, 76
The final array after sorting will be: [3, 5, 6, 12, 23, 24, 35, 53, 76]
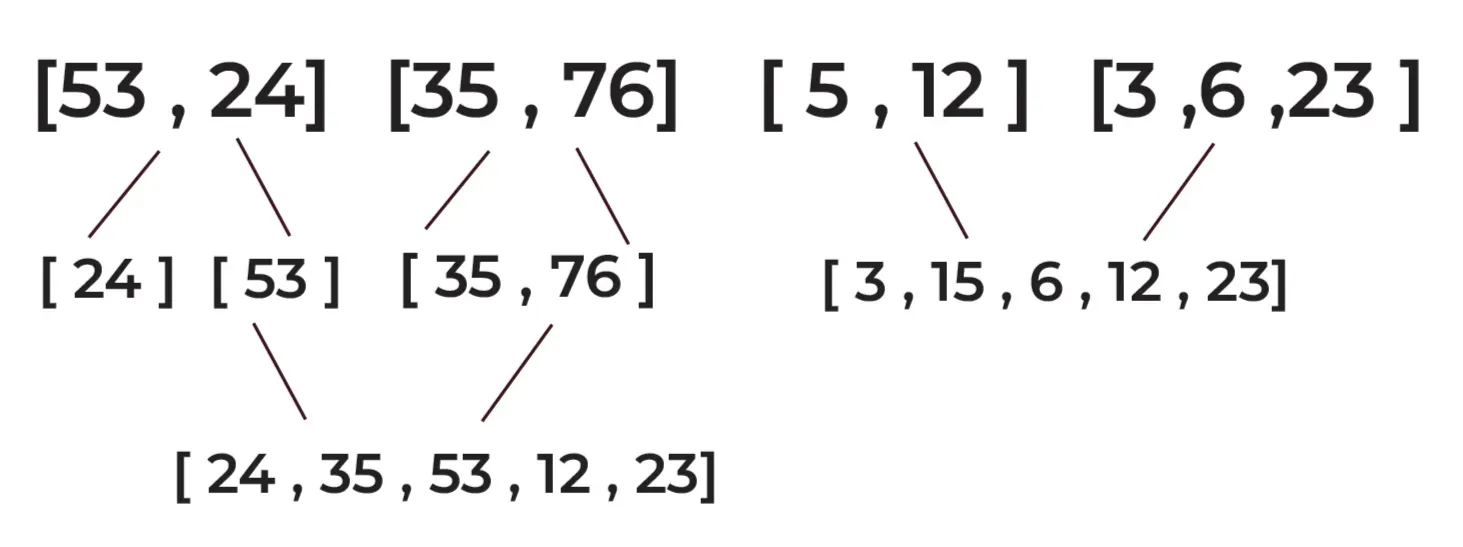
That’s how the merge sort algorithm works. Let’s see the code example for implementing the merge sort algorithm in the C program.
Pseudocode
Let’s see the pseudocode for the implementation of merge sort using a recursive approach.
mergeSort(arr[], left, right)
If left < right
- Find the middle point to divide the array into two halves:
mid = (left + right) / 2
- Call MergeSort for the first half:
mergeSort(arr, left, mid)
- Call MergeSort for the second half:
mergeSort(arr, mid + 1, right)
- Merge the two halves sorted above:
mergeTwoHalves(arr, left, mid, right)
mergeTwoHalves(arr[], left, mid, right)
n1 = mid – left + 1
n2 = right – mid
Create temp arrays t1[n1] and t2[n2]
Copy data to temp arrays t1[] and t2[]
Merge the temp arrays back into arr[left..right]
Copy the remaining elements of t1[], if any
Copy the remaining elements of t2[], if any
Explanation:
In this pseudocode, we have defined two functions. The mergeSort() function is responsible for dividing the array elements into sub-arrays or halves for sorting, whereas the mergeTwoHalves() is responsible for merging the two split arrays in temporary arrays and converting them again to the original array.
The mergeTwoHalves() is a significant function as it performs the merging of two sorted sub-arrays, to build a single sorted array.
Example: