For Java developers, Spring Boot is a crucial framework that makes it easier to make stand-alone and production-grade spring-based applications. Since it is one of the most popular topics in job interviews for Java-related jobs, mastery of Spring Boot indicates that one can quickly develop and deploy reliable applications.
In this blog post, we will cover top 50 interview questions on spring boot with answers to help you get ready. We will look at basic, intermediate and advanced questions so as to ensure we have covered everything. Regardless of whether you are just starting out or an experienced developer, this guide will give you the knowledge that you need to ace your next Spring Boot interview.
Basic-level Spring Boot Interview Questions
1. What is Spring Boot?
Spring Boot is a framework that simplifies developing spring-based applications. It comes with pre-configured templates and tooling that reduce setup time, enabling developers to produce standalone production-ready applications with minimal configuration. Traditional spring apps required a lot of boilerplate code and configuration, but this was removed.
2. Explain the Features of Spring Boot.
Spring Boot provides several features that make it a very strong application framework:
- Auto-Configuration: Set up your spring application automatically based on the jar dependencies added to your project.
- Standalone Applications: Allows for the development of non-web applications without having to rely on an external app server.
- Production-Ready: Features such as health check-ups, metrics and externalised configuration are included in order to prepare them for production use.
- Embedded Servers: Embedded servers like Tomcat, Jetty, and Undertow simplify deployment
- Spring Boot CLI: A command line tool for running Groovy scripts that gives quick-start support when developing in Java’s favourite JVM language
3. What are the Advantages of Using Spring Boot?
Using Spring Boot provides several advantages:
- Reduced Development Time: Simplifies configuration and setup, reducing development time.
- Embedded Servers: Eliminates the need to deploy WAR files, as embedded servers like Tomcat are included.
- Microservices Support: Ideal for developing microservices with its lightweight and modular approach.
- Production-Ready: Built-in monitoring and management capabilities make applications ready for production.
- Community and Support: A large community and extensive documentation provide robust support and resources.
4. Can We Create a Non-Web Application in Spring Boot?
Yes, Spring Boot can be used to create non-web applications. Spring Boot is not limited to web applications; it can be used for a variety of application types, including batch processing, command-line applications, and more.
5. What are the Key Components of Spring Boot?
The key components of Spring Boot include:
- Spring Boot Starter: Provides a set of convenient dependency descriptors to include various Spring modules.
- Spring Boot Auto-Configuration: Automatically configures the application based on the included dependencies.
- Spring Boot CLI: A command-line tool for developing Spring applications.
- Spring Boot Actuator: Adds production-ready features like monitoring and metrics.
- Embedded Server: Includes embedded servers like Tomcat, Jetty, and Undertow.
6. Explain How to Create a Spring Boot Application Using Maven.
Creating a Spring Boot application using Maven involves the following steps:
- Setup Maven: Ensure Maven is installed on your system.
- Create Project: Use the following command to create a new Maven project:
mvn archetype:generate -DgroupId=com.example -DartifactId=spring-boot-app -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
- Add Dependencies: Update the pom.xml file with Spring Boot dependencies:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
- Create Main Class: Create a main class with @SpringBootApplication annotation:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
- Run Application: Use Maven to run your application:
mvn spring-boot:run
7. Why Do We Prefer Spring Boot Over Spring?
Spring Boot is preferred over traditional Spring for several reasons:
- Simplified Configuration: Reduces boilerplate code and configuration complexity.
- Embedded Servers: Eliminates the need for separate application servers.
- Quick Setup: Allows rapid development with auto-configuration and starter templates.
- Microservices Ready: Ideal for building microservices due to its lightweight nature.
- Production-Ready: Comes with built-in monitoring and management tools.
- Less Code: Requires less code to achieve the same functionality, enhancing productivity.
Feature |
Spring |
Spring Boot |
Configuration |
Manual |
Auto-Configuration |
Server Deployment |
Requires External Server |
Embedded Server |
Setup Time |
Longer |
Quick |
Microservices |
Less Suitable |
Ideal |
Production Tools |
Manual Setup |
Built-in Actuator |
8. Explain the Internal Workings of Spring Boot.
Spring Boot simplifies application development by auto-configuring beans based on the classpath, including embedded servers, and providing production-ready features. It uses Spring Boot Starters to bundle dependencies and the Spring Boot Actuator for monitoring and metrics.
9. How Do You Enable HTTP/2 Support in Spring Boot?
To enable HTTP/2 in Spring Boot:
- Add Dependencies: Ensure necessary dependencies are in pom.xml.
- Configure Properties: Add server.http 2.enabled=true to application.properties.
- SSL Configuration: Ensure SSL is set up, as HTTP/2 requires it.
10. What are the Spring Boot Starter Dependencies?
Spring Boot Starters are pre-defined dependency sets for various functionalities:
- spring-boot-starter-web: Web applications.
- spring-boot-starter-data-jpa: JPA and Hibernate.
- spring-boot-starter-test:
- spring-boot-starter-security:
- spring-boot-starter-thymeleaf: Thymeleaf templating.
11. How Does a Spring Application Get Started?
A spring application starts with a main method called SpringApplication.run(). This sets up the default configuration, starts the Spring application context, performs a classpath scan, and starts the embedded server if it’s a web application.
12. What is Spring Boot Dependency Management?
Spring Boot Dependency Management uses a BOM (Bill of Materials) to manage dependency versions. By relying on Spring Boot’s BOM, you can declare dependencies without specifying versions, ensuring compatibility and consistency.
13. What Does the @SpringBootApplication Annotation Do Internally?
@SpringBootApplication combines:
- @Configuration: Defines bean configurations.
- @EnableAutoConfiguration: Enables auto-configuration.
- @ComponentScan: Scans for components in the package.
This annotation simplifies the configuration process.
14. Mention the Minimum Requirements for a Spring Boot System.
The minimum requirements for a Spring Boot system include:
- JDK: Compatible version (JDK 8 or higher).
- Build Tool: Maven or Gradle.
- IDE: Recommended for ease of development.
- Hardware: Basic hardware with at least 2GB RAM and a modern CPU.
15. What is Spring Initializr?
Spring Initializr is a web-based tool that simplifies the creation of Spring Boot projects. It provides a user-friendly interface to generate a Maven or Gradle project structure with the necessary dependencies and configurations.
16. What are Spring Boot CLI and the Most Used CLI Commands?
Spring Boot CLI is a command-line tool that allows you to quickly create, run, and test Spring applications using Groovy scripts. It simplifies the development process by reducing boilerplate code and configuration.
Most Used CLI Commands
Command |
Description |
spring init |
Creates a new project with default settings. |
spring run <file.groovy> |
Runs a Groovy script. |
spring test <file.groovy> |
Runs test in a Groovy script. |
spring install <dependency> |
Installs a specified dependency. |
17. How Can We Create a Custom Endpoint in Spring Boot Actuator?
To create a custom endpoint in Spring Boot Actuator, follow these steps:
- Add Dependency: Ensure Actuator is included in your pom.xml:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
@RestController
public class CustomEndpoint {
@GetMapping(“/custom-endpoint”)
public String customEndpoint() {
return “Custom Endpoint Response”;
}
}
- Expose Endpoint: Add the endpoint to the Actuator properties in application.properties:
management.endpoints.web.exposure.include=custom-endpoint
18. Explain Spring Data.
Spring Data is a part of the Spring framework that simplifies data access by providing a unified and consistent data access approach. It supports various data storage technologies, such as relational databases, NoSQL databases, and cloud-based data services. The key components of Spring Data include:
- Spring Data JPA: For relational databases using JPA and Hibernate.
- Spring Data MongoDB: For MongoDB.
- Spring Data Redis: For Redis.
- Spring Data Elasticsearch: For Elasticsearch.
19. Explain How to Register a Custom Auto-Configuration.
To register a custom auto-configuration in Spring Boot, follow these steps:
- Create Configuration Class:
@Configuration
public class CustomAutoConfiguration {
@Bean
public MyService myService() {
return new MyService();
}
}
- Create META-INF/spring.factories: Create a spring.factories file under src/main/resources/META-INF/ with the following content:
org.springframework.boot.autoconfigure.EnableAutoConfiguration=
com.example.demo.CustomAutoConfiguration
This setup ensures that Spring Boot will automatically configure MyService when the application starts.
20. What Are the Steps to Follow to Add Custom JS Code with Spring Boot?
To add custom JS code to a Spring Boot application, follow these steps:
- Create Static Directory: Place your JavaScript files in the src/main/resources/static directory.
- Add HTML Template: Reference your JavaScript files in your HTML template, typically located in src/main/resources/templates.
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<title>Custom JS Example</title>
<script src=”/static/js/custom.js”></script>
</head>
<body>
<h1>Hello, Spring Boot!</h1>
</body>
</html>
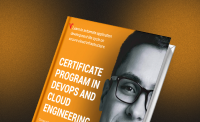
Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
21. What are the steps to connect an external database, such as MySQL or Oracle?
To connect an external database like MySQL or Oracle to a Spring Boot application, follow these steps:
- Add Dependencies: Include the necessary database driver in your pom.xml:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!– For Oracle, use the following –>
<!– <dependency>
<groupId>com.oracle.database.jdbc</groupId>
<artifactId>ojdbc8</artifactId>
</dependency> –>
- Configure Database Properties: Add your database connection properties in application.properties:
spring.datasource.url=jdbc:mysql://localhost:3306/yourdatabase
spring.datasource.username=yourusername
spring.datasource.password=yourpassword
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
- Create a Repository: Define a Spring Data repository to interact with your database:
@Repository
public interface UserRepository extends JpaRepository<User, Long> {}
22. What are the Basic Spring Boot Annotations?
Basic Spring Boot annotations include:
- @SpringBootApplication: Combines @Configuration, @EnableAutoConfiguration, and @ComponentScan.
- @RestController: Indicates that the class is a RESTful web service controller.
- @RequestMapping: Maps HTTP requests to handler methods.
- @Autowired: Automatically injects dependencies.
- @Entity: Specifies that the class is an entity and is mapped to a database table.
- @Service: Marks a class as a service provider.
23. What is Spring Boot Dependency Management?
Spring Boot Dependency Management simplifies managing dependencies by using a centralised BOM (Bill of Materials). It ensures compatible versions of dependencies by allowing you to declare them without specifying versions, leveraging Spring Boot’s predefined versions.
24. Can We Change the Port of the Embedded Tomcat Server in Spring Boot?
Yes, you can change the port of the embedded Tomcat server in Spring Boot by adding the following configuration to application.properties:
server.port=8081
This changes the default port from 8080 to 8081.
25. What is the Starter Dependency of the Spring Boot Module?
The starter dependency of a Spring Boot module simplifies and includes a group of related dependencies. For example, spring-boot-starter-web includes dependencies for building web applications using Spring MVC, embedded Tomcat, and more:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
26. What is Spring Boot CLI, and How Can the Spring Boot Project Be Executed Using Boot CLI?
Spring Boot CLI is a command-line tool that simplifies the development of Spring applications using Groovy scripts. It reduces boilerplate code and allows for quick prototyping.
Steps to Execute a Spring Boot Project using CLI:
@RestController
class Example {
@RequestMapping(“/”)
String home() {
“Hello, Spring Boot CLI”
}
}
- Run the Script: Use the CLI to run the script:
spring run app.groovy
27. Can We Disable the Default Web Server in the Spring Boot Application?
Yes, you can disable the default web server in a Spring Boot application by setting the following property in application.properties:
spring.main.web-application-type=none
28. How Do You Disable a Specific Auto-Configuration Class?
To disable a specific auto-configuration class, use the @SpringBootApplication annotation with the exclude attribute:
@SpringBootApplication(exclude = {DataSourceAutoConfiguration.class})
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
29. Can We Create a Non-Web Application in Spring Boot?
Yes, you can create a non-web application in Spring Boot. Set spring.main.web-application-type=none in application.properties and ensure your main class does not start an embedded server.
30. Describe the Flow of HTTPS Requests Through the Spring Boot Application.
- HTTPS Request: A client sends an HTTPS request to the server.
- SSL Handshake: The server and client perform an SSL handshake to establish a secure connection.
- DispatcherServlet: The request is forwarded to the DispatcherServlet.
- Handler Mapping: The DispatcherServlet maps the request to the appropriate handler (controller).
- Controller Processing: The controller processes the request and generates a response.
- Response: The DispatcherServlet sends the response back to the client over the secure connection.
31. Explain @RestController Annotation in Spring Boot.
@RestController is a specialised version of @Controller. It combines @Controller and @ResponseBody, indicating that the controller’s methods return data directly (usually JSON) instead of rendering a view.
32. What are the Steps to Deploy Spring Boot Web Applications as JAR and WAR Files?
Deploying as JAR:
- Build Project: Package the application using Maven or Gradle:
mvn clean package
- Run JAR: Execute the JAR file:
java -jar target/your-application.jar
Deploying as WAR:
- Change Packaging: Update pom.xml to war packaging:
<packaging>war</packaging>
- Extend SpringBootServletInitializer: Modify the main application class:
@SpringBootApplication
public class Application extends SpringBootServletInitializer {
@Override
protected SpringApplicationBuilder configure(SpringApplicationBuilder application) {
return application.sources(Application.class);
}
}
- Build WAR: Package the application:
mvn clean package
- Deploy WAR: Deploy the WAR file to an external server like Tomcat.
33. What is the Difference Between @RequestMapping and @GetMapping?
Annotation |
Purpose |
HTTP Method |
@RequestMapping |
General-purpose mapping for all HTTP methods |
GET, POST, etc. |
@GetMapping |
Specific mapping for GET requests |
GET |
34. Difference Between @Controller and @RestController.
Annotation |
Purpose |
Return Type |
@Controller |
Handles traditional web MVC controllers |
Views (e.g., HTML) |
@RestController |
Handles RESTful web services |
Data (e.g., JSON) |
35. Explain the Differences Between @SpringBootApplication and @EnableAutoConfiguration.
Annotation |
Purpose |
Combined Annotations |
@SpringBootApplication |
Simplifies configuration of Spring Boot |
@Configuration, @EnableAutoConfiguration, @ComponentScan |
@EnableAutoConfiguration |
Enables Spring Boot’s auto-configuration |
N/A |
36. What are Profiles in Spring?
Profiles in Spring allow you to define different configurations for different environments (e.g., development, production). You can activate a profile by setting the spring.profiles.active property in application.properties or as a command-line argument.
37. Mention the Differences Between WAR and Embedded Containers.
Deployment Type |
Description |
Usage |
WAR File |
Requires deployment to an external application server |
Traditional enterprise apps |
Embedded Container |
Includes an embedded server, runs as a standalone JAR |
Microservices and simple deployments |
Advanced-level Spring Boot Interview Questions
38. What is a Spring Boot Actuator?
Spring Boot Actuator provides production-ready features to help you monitor and manage your application. It includes several built-in endpoints that expose information about the application’s health, metrics, info, dump, env, and more.
39. How Do You Enable Actuator in the Spring Boot Application?
To enable Actuator in a Spring Boot application, add the following dependency to your pom.xml:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Then, configure the desired endpoints in application.properties:
management.endpoints.web.exposure.include=*
40. What is the Purpose of Using @ComponentScan in the Class Files?
@ComponentScan is used to specify the packages to scan for annotated components. This helps Spring to detect and register beans with annotations like @Component, @Service, @Repository, and @Controller automatically.
41. What are the @RequestMapping and @RestController Annotations in Spring Boot Used For?
Annotation |
Purpose |
Usage |
@RequestMapping |
Maps HTTP requests to handler methods |
Used on methods or classes |
@RestController |
Simplifies creating RESTful web services |
Combines @Controller and @ResponseBody |
42. How Do You Get the List of All the Beans in Your Spring Boot Application?
You can get the list of all beans in a Spring Boot application by using the ApplicationContext:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class BeanController {
@Autowired
private ApplicationContext applicationContext;
@GetMapping(“/beans”)
public String[] getBeans() {
return applicationContext.getBeanDefinitionNames();
}
}
43. In Which Layer Should the Boundary of a Transaction Start?
The boundary of a transaction should start at the service layer. This is where the business logic resides, and transactions are managed.
44. Can We Check the Environmental Properties in Your Spring Boot Application and Explain How?
Yes, you can check environmental properties using the Environment object:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.env.Environment;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class EnvController {
@Autowired
private Environment env;
@GetMapping(“/env”)
public String getEnvProperty() {
return env.getProperty(“spring.application.name”);
}
}
45. How Do You Enable the Debugging Log in the Spring Boot Application?
To enable debugging logs, add the following property in application.properties:
logging.level.org.springframework=DEBUG
This sets the log level to DEBUG for the Spring framework.
46. What is Dependency Injection and Its Types?
Dependency Injection (DI) is a design pattern that allows a class to receive its dependencies from an external source rather than creating them itself.
Type |
Description |
Constructor Injection |
Dependencies are provided through the class constructor |
Setter Injection |
Dependencies are provided through setter methods |
Field Injection |
Dependencies are injected directly into the fields |
47. What is an IOC Container?
The Inversion of Control (IOC) container is responsible for managing the lifecycle and configuration of application objects. It creates, configures, and manages beans, and handles their dependencies.
48. What is the Need for Spring Boot DevTools?
Spring Boot DevTools improves the development experience by providing features like automatic restart, live reload, and configurations for faster feedback during development.
49. What is the Difference Between Constructor and Setter Injection?
Injection Type |
Description |
Pros |
Cons |
Constructor Injection |
Dependencies are provided via constructor |
Ensures immutability, promotes mandatory dependencies |
Harder to handle optional dependencies |
Setter Injection |
Dependencies are provided via setter methods |
Useful for optional dependencies, more flexible |
Can lead to inconsistent state if not handled properly |
50. What is Thymeleaf?
Thymeleaf is a contemporary server-side Java template engine for web and standalone environments. It processes HTML, XML, JavaScript, CSS and plain text files perfectly well which does not require any additional steps using other technologies. Thymeleaf can be used with Spring, however it is designed to be friendly for natural templating which makes it easier to create templates that work well in web browsers.
Conclusion
Spring boot simplifies the development and deployment of Java applications. This framework has a number of features such as auto-configuration, embedded servers and Actuator for monitoring which would improve developers’ productivity and efficiency. Essential concepts for mastering Spring Boot include DI, @RestController, @RequestMapping annotations etc., as well as transaction management and configuration customization.
With tools such as Spring Boot CLI and Spring Boot DevTools, developers can streamline their workflow and concentrate on writing robust, scalable applications. Regardless of whether you are developing web-based or non-web based applications, Spring Boot provides a versatile solution for modern-day development requirements.