Python data structures are the superheroes of coding. You’ve got lists, the multitaskers; imagine juggling a mix of numbers, words, and even more lists effortlessly. Then come sets, the guardians of uniqueness; no twins allowed in this exclusive club! Tuples? They’re the steady rock, unchanging and dependable.
These structures are like a toolbox filled with gadgets, each one perfect for specific tasks. Need to find something super fast? Sets are your speed demons. Want to keep things neat and tidy? Hello, dictionaries! And when do you want both order and flexibility? Lists and tuples are your trusty sidekicks.
The best part? Python is like a cool playground where you get to experiment with these structures. Its friendly nature and endless possibilities make it the perfect canvas for your coding adventures.
So, buckle up, fellow adventurers! Get ready to wield these magical structures, craft clever programs, and unravel the mysteries of Python coding like never before. Let’s embark on this thrilling journey together and conquer the code-scape with Python data structures!
What Are Data Structures?
A data structure is the architectural backbone of how information is arranged and stored within a computer’s memory using a programming language. This organisation isn’t just about storing data; it’s about optimising how that data is accessed, modified, and managed. Think of it as the framework that enables efficient handling of information within a computer system.
The significance of data structures lies in their role as foundational elements within software development. These structures form the core building blocks upon which modern software applications are constructed. They provide the scaffolding for organising, managing, and manipulating data effectively, allowing programmers to create efficient and responsive systems.
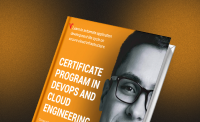
Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
What Are Python Data Structures?
In Python, the core data structures can be categorised into two main types: mutable and immutable. Understanding these structures is crucial as they form the foundation for organising and manipulating data within Python programs.
Mutable data structures, as the name suggests, allow modification after their creation. Python offers three primary mutable data structures: lists, dictionaries, and sets. These structures provide flexibility by enabling additions, removals, and alterations to their elements.
What is DSA in Python?
DSA, Data Structures and Algorithms within Python form a formidable partnership, collaborating seamlessly to tackle problems with efficiency. Python’s inherent simplicity and adaptability render it an ideal language for both implementing and exploring diverse data structures and algorithms. Be it delving into sorting algorithms such as quicksort or delving deep into data structures like stacks, queues, trees, or graphs, Python furnishes an extensive platform for exploration and experimentation.
Python Data Structures – Lists
In Python, lists serve as dynamic mutable arrays, enabling the storage of an ordered collection of items. Unlike arrays in many other programming languages, Python’s lists aren’t restricted to containing elements of the same data type; they can encompass a variety of data types and objects, such as integers, strings, functions, and more.
One defining feature of lists is their ordered nature. They retain the sequence in which elements are inserted, allowing access through integer indices. This indexing starts from 0, enabling specific element retrieval based on its position within the list.
Manipulating lists is intuitive and flexible. Methods like .append() facilitate the addition of new elements, while.remove() selectively eliminates elements from a list. Moreover, direct access to list elements by index allows for modifications, enabling the replacement of elements as needed. The Python documentation provides an exhaustive list of methods available for list manipulation.
A standout attribute of lists is their dynamic nature. Unlike some data structures, lists don’t require specifying the number of elements during creation. They can expand or shrink dynamically, offering adaptability as data demands change.
Lists shine when there’s a need to store a heterogeneous collection of data types, facilitating subsequent operations like addition, removal, or iteration through elements via looping. Their versatility extends to housing other data structures, allowing the creation of nested structures like lists of dictionaries, tuples, or even lists themselves. For instance, a table can be represented as a list of lists, where each inner list corresponds to a column in the table—a common practice in data analysis workflows.
Pros of Lists:
- Flexible Storage: Easy way to store a diverse collection of related objects.
- Simple Modification: Simple addition, removal, and alteration of elements.
- Nested Structures: Suitable for creating complex nested data structures.
Cons of Lists:
- Performance Impact: Slower performance in arithmetic operations compared to specialised structures like NumPy arrays.
- Disk Space Utilisation: Occupies more disk space due to its internal implementation.
Python Data Structures – Tuples
Tuples in Python closely resemble lists as they both contain an ordered collection of elements. However, the key distinction lies in their immutability; tuples, once created, cannot be modified. This property makes tuples an ideal choice when a data structure is needed, ensuring its contents remain unchanged after creation.
One notable advantage of tuples is their suitability as dictionary keys, provided all elements within the tuple are immutable. This characteristic allows tuples to serve as reliable keys within dictionaries.
Creating tuples is straightforward; they can be formed using round brackets () or the tuple() constructor. Furthermore, effortless conversion between lists and tuples is possible, offering flexibility in data structure transformations. For instance, conversion from a tuple to a list (or vice versa) can be performed seamlessly using appropriate Python syntax.
Pros of Tuples:
- Immutable Nature: Once created, their contents remain fixed, preventing inadvertent modifications.
- Usable as Dictionary Keys: When all elements are immutable, tuples serve effectively as keys within dictionaries.
Cons of Tuples:
- Incompatibility with Modifiable Objects: Unsuitable for situations involving modifiable objects; lists are preferred in such scenarios.
- Immutability Limits Modification: The inability to modify tuples restricts their usage in dynamic contexts.
- Memory Occupancy: Tuples tend to occupy more memory compared to lists, impacting memory utilisation.
Despite their immutability, tuples serve as robust data structures, offering stability and reliability in situations where data integrity is paramount. However, their fixed nature may limit their applicability in scenarios requiring dynamic modifications or the use of mutable objects.
Python Data Structures – Sets
In Python, sets stand as mutable and dynamic collections comprising unique, immutable elements. These sets significantly diverge from lists despite apparent similarities.
Sets enforce uniqueness, allowing only distinct elements to be stored. This unique trait makes sets an ideal tool for eliminating duplicates from lists. Much like their mathematical counterparts, sets offer a range of unique operations such as union, intersection, and more. Additionally, they excel in swiftly verifying the presence of specific elements within the collection, showcasing high efficiency.
Pros of Sets:
- Unique Operations: Sets support distinctive operations that set them apart from other structures, offering functionalities like set unions and intersections.
- Efficiency in Element Checks: Sets boast exceptional speed when verifying whether a particular element is present within the collection.
Cons of Sets:
- Intrinsic Unordered Nature: Sets lack inherent ordering, which may be problematic if preserving the insertion order is crucial.
- Limited Element Modification: Unlike lists, sets do not support altering elements via indexing, restricting the modification capabilities.
Sets serve as powerful tools when uniqueness and rapid element checks are pivotal. Their efficiency in handling unique operations and swift element checks makes them a valuable asset in scenarios where these functionalities are critical.
However, their inherent unordered nature and the inability to modify elements via indexing can pose limitations, particularly in situations where preservation of insertion order or direct element manipulation is required.
Utilising sets effectively involves recognising their unique strengths and limitations, allowing for informed decisions when choosing the appropriate data structure to optimise operations and meet specific programming needs.
Why Are Tuples Preferred Over Lists?
Tuples present a compelling choice when data immutability and efficiency in program execution are priorities. Their immutability ensures data integrity by preventing inadvertent modifications, making them the preferred option in scenarios where data integrity and stability are crucial.
One significant advantage of tuples lies in their immutability. By design, tuples cannot be altered once created, safeguarding the data against accidental additions, modifications, or removals. This property proves invaluable when creating objects intended to remain unaltered throughout their lifespan.
Moreover, tuples are more memory-efficient compared to lists. Their memory footprint is smaller, contributing to reduced memory usage within programs. Additionally, tuples enhance program execution speed when compared to lists. Lists tend to be slower due to the creation of new objects with every execution, whereas tuples, identified by Python as one immutable object, are constructed as a single entity.
This difference in the underlying handling of lists and tuples translates into improved performance with tuples, as they are treated as a single immutable unit rather than being subject to object creation each time they’re accessed or manipulated.
Advantages of Tuples Over Lists:
- Immutability Ensures Data Integrity: Tuples prevent accidental modifications, ensuring data remains intact and consistent.
- Memory Efficiency: Tuples use less memory compared to lists, contributing to better memory utilisation within programs.
- Enhanced Program Execution Speed: Tuples are faster due to their identification as a single immutable object, avoiding the overhead associated with creating new objects, unlike lists.
Tuples, with their immutable nature and efficiency benefits, serve as a robust choice in scenarios where data stability, reduced memory consumption, and optimised program execution are paramount. Leveraging tuples appropriately can significantly enhance the reliability and performance of Python programs.
Long Story Short
Mastering Python’s diverse data structures unlocks a world of possibilities for any aspiring coder. Understanding how lists, tuples, sets, dictionaries, and more operate is not just about manipulating data; it’s about crafting efficient, scalable solutions that form the backbone of robust programs.
Hero Vired is committed to empowering learners to harness the full potential of Python’s data structures. Its comprehensive courses delve deep into these structures, offering hands-on experiences, real-world applications, and expert guidance. Whether you’re diving into data analysis, optimising program performance, or crafting innovative solutions, these courses equip you with the skills and knowledge needed to excel in a dynamic tech landscape.
Join new-age courses at Hero Vired and start on a transformative learning journey. Unravel the magic of Python’s data structures together and pave the way for a successful tech career.
FAQs
Python's fundamental data structures comprise lists, sets, tuples, and dictionaries. Each structure possesses its distinct characteristics. These data structures act as "containers," arranging and categorising data by type. Their dissimilarities arise from variations in mutability and arrangement.
Python's straightforward and easily understood syntax proves beneficial for newcomers, enabling a focus on algorithm design over intricate language intricacies. Python boasts an extensive community and an abundance of Python libraries like NumPy and pandas, simplifying the implementation of DSA. Python's most adaptable data structure is the list, serving as a versatile container capable of storing diverse data items such as integers, strings, or even nested lists. Lists exhibit mutability, enabling alterations to their elements post-creation.
A module in Python refers to a file marked with the ".py" extension, housing Python code that can be utilised by importing it into another Python program. Essentially, modules can be likened to code libraries or files encompassing a collection of functions meant to be incorporated within your application.
Updated on August 30, 2024