Memory management is a very important concept in computer operating systems. Paging and Segmentation are two powerful techniques for memory management in operating systems. A multiprogramming computer uses some memory for the operating system and uses other memory for other processes. Memory management must allocate memory to the different processes.
In this article, we will see the difference between paging and segmentation for memory management. We will learn about the features, advantages, and disadvantages of both memory management techniques, paging and segmentation. But before we move on to learning about paging and segmentation, we need to have an understanding of memory management like types and more. So, let’s get started!
What is Memory Management?
Memory management is the process of allocating sections of computer memory, known as blocks, to different operating programs to maximise system performance. Memory management works like when the program comes into the RAM, it must be capable of holding more and more processes. When we increase the number of programs, the RAM or memory must be capable of handling a large number of programs. Therefore, to handle more programs in the memory, we use a technique known as the partitioning method. In the partitioning method, we accommodate an increasing number of programs by creating memory partitions and also partitioning the program itself to store it in the memory.
Paging and Segmentation are two techniques for memory management that help in accommodating more and more programs into the memory without any failure. Both these techniques are currently being used in operating systems for efficient memory management. The system can allocate memory more effectively by using paging and segmentation, but they work differently and are better suited for different kinds of tasks.
In an operating system (OS), there are two types of memory management techniques or allocation:
1. Contiguous memory allocation
In a contiguous memory allocation, the partition blocks are created and stored as a contiguous array of blocks after partitioning. In this, the programs are allocated the continuous memory space in the main memory. The contiguous memory allocation is of two types: Fixed continuous partitioning and Variable continuous partitioning.
2. Non-contiguous memory allocation
In a non-contiguous memory allocation, the partition blocks are created and stored in a non-contiguous location after partitioning. The programs may be stored at different locations in the memory but not contiguous. The non-contiguous memory allocation is of two types: Paging and Segmentation.
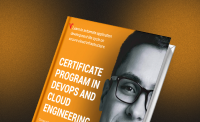

Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
What is Paging?
Paging is a memory management technique of the OS in which the physical memory is divided into the fixed size pages, and the main memory is divided into fixed size frames. The fixed-size frames are also known as page frames. The size of each page will be equal to the size of each page frame.
The basic goal of paging is to remove the requirement for memory to be allocated in a contiguous manner. By using paging, the OS can break programs into small fixed-size pages that are kept in non-contiguous locations (like a linked list, tree form, etc.) in physical memory, as opposed to allocating memory in contiguous chunks.
In paging, if there are N number of pages then there will be N number of page frames, and each page frame will accommodate each page into it. For example, if a program arrives in the memory, the size of the program will be determined by the CPU. Now, suppose the incoming program is divided into 3 pages by the CPU, then there must be at least 3-page frames free available inside the main memory.
If all 3 page frames are available, each page will be allocated to the page frame. Like, the program’s initial page is loaded into one of the allotted frames, and during this operation, the frame number is added to the page table. The frame number of the subsequent page is loaded to the page table, it is loaded into an additional frame, and so on.
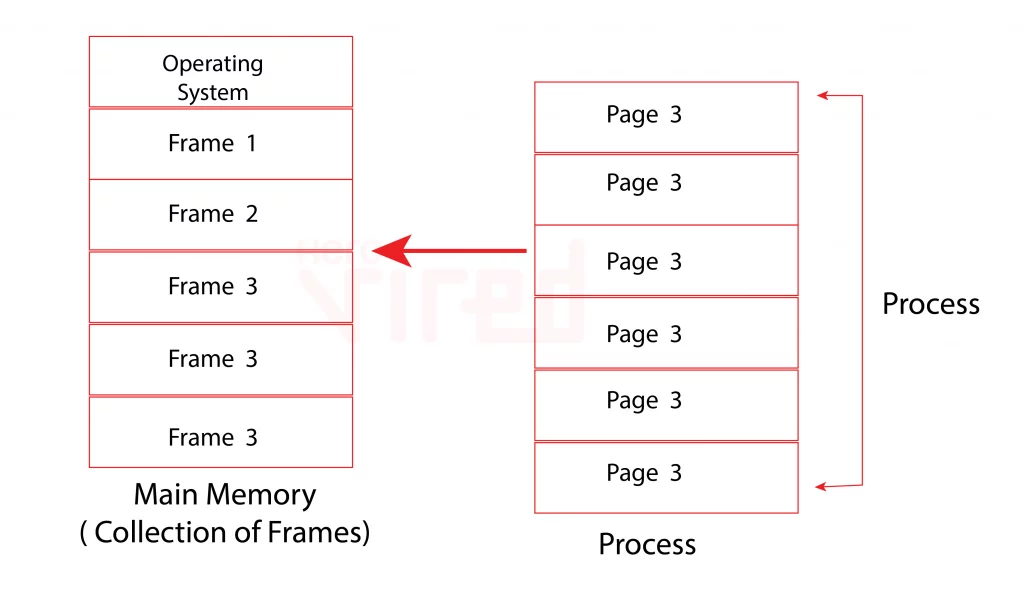
Features
- Paging divides the memory into fixed-size blocks known as pages.
- For easy mapping between pages and frames, the physical memory is partitioned into frames that are the same size as pages.
- Because each page can have its rights, the operating system can manage which memory regions are accessible to which users. It finds and stops any unauthorised entry.
- Every process keeps track of the mapping between its virtual pages and the actual memory frames in a page table.
- Paging translates the virtual address into a physical address.
- When physical memory is full, the system must replace current pages with new ones. To identify which pages to replace, a variety of algorithms are utilised, including Least Recently utilised (LRU), First-In-First-Out (FIFO), and Optimal Page Replacement.
- Demand paging loads pages into memory only when they are needed, lowering overall memory utilisation and increasing efficiency.
Advantages of Paging
- Paging reduces the chances of external fragmentation.
- Paging allows the efficient usage of memory in computer systems.
- Fixed-size blocks called pages are created to reduce the wastage of memory and allocation of memory in uniform blocks.
- Paging provides isolation between processes by ensuring that each process has its page table.
- Paging ensures security and stability by preventing one process from accessing another’s memory.
- Paging allows for demand paging in memory management.
- Easy swapping or replacing of processes between pages and frames.
Disadvantages of Paging
- Paging can issue internal fragmentation as some memory may remain unused due to fixed-size block allocation.
- Overhead issues due to the creation of page tables.
- Paging requires a large amount of memory consumption and processing power.
- It may cause page faults when the required page is not available in the memory.
Example of Paging:
//import statements
#include <stdio.h>
#include <stdlib.h>
// defaults
#define SIZE_OF_PAGE 1024 // 1 KB
#define SIZE_OF_MEMORY 32640 // 32 KB
#define NUMBER_PAGES (SIZE_OF_MEMORY / SIZE_OF_PAGE)
// define a struct for frame number and valid
typedef struct {
int fNumber;
int valid;
} PageEntry;
PageEntry pTable[NUMBER_PAGES];
// Simulate physical memory as an array
char phMemory[SIZE_OF_MEMORY];
void createPageTable() {
for (int i = 0; i < NUMBER_PAGES; i++) {
pTable[i].valid = 0; // initially mark all pages as invalid
}
}
// define the physical address using logical address
int getPhysicalAddress(int logAddress) {
int pNumber = logAddress / SIZE_OF_PAGE;
int offset = logAddress % SIZE_OF_PAGE;
if (pTable[pNumber].valid) {
return pTable[pNumber].fNumber * SIZE_OF_PAGE + offset;
} else {
printf(“Page fault occurred on page: %dn”, pNumber);
// Handle page fault (load the page into memory)
// For simplicity, let’s assume each page maps to the same frame number
pTable[pNumber].fNumber = pNumber;
pTable[pNumber].valid = 1;
return pTable[pNumber].fNumber * SIZE_OF_PAGE + offset;
}
}
// read operation and print the physical address
void readMemory(int logAddress) {
int phAddress = getPhysicalAddress(logAddress);
printf(“Reading from physical address: %dn”, phAddress);
}
// Main function
int main() {
// function call
createPageTable();
int logAddress = 1024; // define the logical address
readMemory(logAddress);
// function call
createPageTable();
int logAddress2 = 2048; // define the logical address
readMemory(logAddress2);
// function call
createPageTable();
int logAddress1 = 3072; // define the logical address
readMemory(logAddress1);
return 0;
}
Output:
Page fault occurred on page: 1
Reading from physical address: 1024
Page fault occurred on page: 2
Reading from physical address: 2048
Page fault occurred on page: 3
Reading from physical address: 3072
Explanation:
In this example, we have created a simple program in C language that demonstrates how paging is done. Here, we have initialised a page table and used paging to emulate a basic memory read operation. Using the page table, the getPhysicalAddress method converts a logical address to a physical address. It then also handles page faults by loading the necessary page into memory.
What is Segmentation?
Segmentation is a non-contiguous memory management technique in OS, in which main memory is divided into small logical units known as segments and stores data like function, procedure, or any data structure. In segmentation, the logical units are created in variable size, unlike paging, where fixed-size blocks are being created.
In terms of a compiler, it automatically divides a program into segments when it is compiled wherein, the different segments may be produced by a compiler for global variables, heaps, stacks, etc. Although segmentation gives you more control over memory management, segments of different sizes may cause fragmentation (internal or external) problems.
For example, there is a program that needs to do a task. Then when the program comes to the CPU, the program is divided into small segments, and each segment will contain parts that execute related functions.
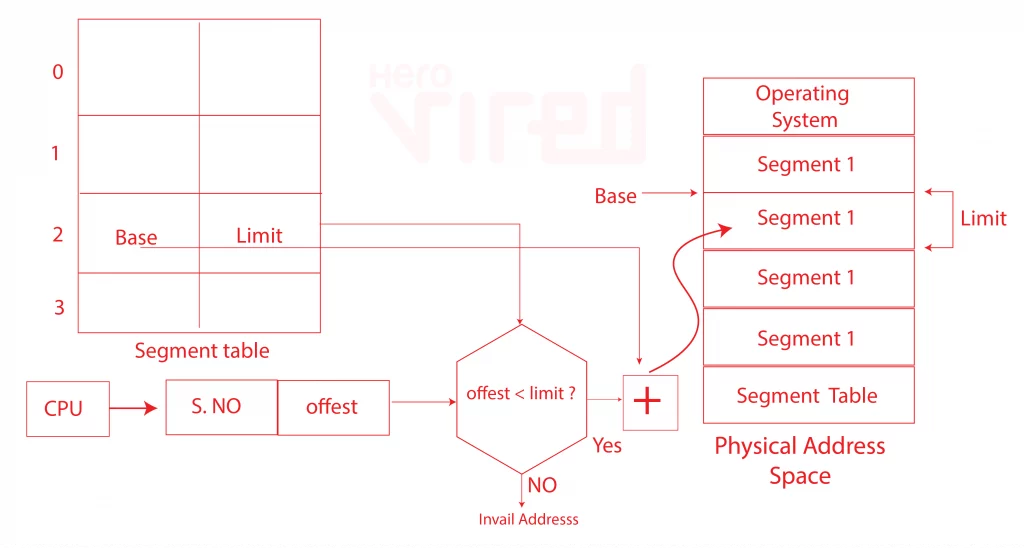
Features
- Segments are created in segmentation as individual logical units.
- Each segment represents a logical unit, such as a function, array, or data structure, providing a more straightforward mapping to the programmer’s perspective on memory.
- A segment table is used by each process containing the base address and the limit i.e., the size of the segment.
- Individual segments can contain the program’s code, data, stack, etc., reflecting their distinct logical uses.
- Segmentation allows the sharing of resources among various processes in the memory.
- Accessing memory outside the defined limits of a segment results in a segmentation fault, enhancing the protection and stability of the system.
- Segmentation reduces the chances of internal fragmentation.
Advantages of Segmentation
- There is no internal fragmentation in segmentation.
- A proper structure of the logical representation of memory is provided in segmentation.
- Easy for programmers to understand the memory view.
- Protection and isolation of different segments can be achieved.
- Unauthorised access can be prevented in segmentation when there is access to segments outside the defined limit in the segment table.
- Efficient and less memory usage due to no internal fragmentation and due to variable size of segments
- Easy sharing of segment’s data and code between multiple processes and therefore multiple processes can access the same region of the memory.
Disadvantages of Segmentation
- Swapping is not recommended because of the variable size of segments.
- External fragmentation can be achieved in segmentation as free memory is divided into small, non-contiguous blocks, which makes it difficult to allocate large contiguous memory segments.
- Difficult memory management as variable size segments are created. The OS has this overhead to keep track of the size and the location of each segment.
- There is an overhead of a segment table that increases the memory consumption and processing power with time.
Example of Segmentation:
//import statements
#include <stdio.h>
#include <stdlib.h>
// default define
#define SEGMENTS_NUMBER 3
//defining the struct for SegmentTable
typedef struct {
int seg_base;
int limit;
} SegmentTable;
SegmentTable segTable[SEGMENTS_NUMBER];
// physical memory as an array
char phyMemory[32640]; // 16 KB
void initSTable() {
segTable[1].seg_base = 4096;
segTable[1].limit = 2048; // 2 KB
segTable[2].seg_base = 6144;
segTable[2].limit = 1024; // 1 KB
}
// Find the physical address
int getPhyAddress(int segNum, int offset) {
if (segNum < 0 || segNum >= SEGMENTS_NUMBER) {
printf(“Invalid segment number %dn”, segNum);
exit(1);
}
if (offset >= 0 && offset < segTable[segNum].limit) {
return segTable[segNum].seg_base + offset;
} else {
printf(“Segmentation fault at segment %d, offset %dn”, segNum, offset);
exit(1);
}
}
// Read from memory
void rMemory(int segNum, int offset) {
int phyAddress = getPhyAddress(segNum, offset);
printf(“Reading from physical address %dn”, phyAddress);
}
// Main function
int main() {
initSTable();
int segNum = 1;
int offset = 1024;
rMemory(segNum, offset);
return 0;
}
Output:
Reading from physical address 5120
Explanation:
In this example, we have implemented a program for segmentation. Here, we have initialised a segment table and performed a read operation in memory using segmentation. We have created a getPhysicalAddress function that translates a segment number and offsets it into a physical address with the help of the segment table. This table helps in finding out the segmentation faults.
Real-World Applications and Examples
Paging in real-world applications:
Operating systems like Windows, Linux, and macOS, all use a memory management technique paging. They use paging methods to effectively handle big address spaces and maximise memory utilisation, such as demand paging and multi-level page tables.
Segmentation in real-world applications:
Segmentation is now less used, but still, it is being used in specific applications like:
- Embedded systems: Segmentation is still practised in embedded systems for their logical organisation of data and securing their features. Because these systems have limited space and memory, they need to have a great memory management technique.
- Real-time systems: Real-time systems like weather systems, ATC systems, etc., use segmentation to ensure deterministic behaviour so that they can protect their critical system components from external attacks.
- Modular Programming: By enabling programmers to independently define and control the memory requirements of various program modules, segmentation promotes modular programming.
Key Differences Between Paging and Segmentation
The given below are some of the key differences between Paging and Segmentation in memory management in OS:
Criteria |
Paging |
Segmentation |
Allocation type |
Paging is a type of non-contiguous allocation of memory. |
Segmentation is also a type of non-contiguous allocation of memory. |
Memory division |
In paging, the memory is divided into fixed-size pages. |
In paging, the memory is divided into variable-size segments. |
Purpose |
The purpose of paging is to manage the physical memory efficiently. |
The purpose of segmentation is for the logical division of a program. |
Done by |
It is done by the operating system. |
It is done by the compiler. |
Address translation |
For translating the addresses, the page tables are created and used. |
For translating the addresses, the segment tables are created and used. |
Fragmentation |
Paging leads to internal fragmentation within pages. |
Segmentation leads to external fragmentation between each segment. |
Overhead |
There is more overhead as a good amount of memory is used. |
There is less overhead as a less amount of memory is used by the segment tables. |
Access Speed |
Due to page faults, the access speed is low. |
Due to fewer access faults, the access speed is faster. |
Protection |
The paging isolates processes with the help of individual page tables. |
Segmentation protects the processes with the help of segment limits. |
Type of unit |
A physical unit consisting of information. |
A logical unit consisting of information. |
Fault handling |
Page faults can occur on missing pages. |
Segment faults can occur when users try to access non-existing or out-of-bound items. |
Tables |
Page tables are created in Paging. |
Segment tables are created in Segmentation. |
Logical address |
Paging has a logical address consisting of page number and page offset. |
Segmentation has a logical address consisting of segment number and segment offset. |
Hiding |
As Paging is done by the OS, therefore it is completely hidden from the user. |
Segmentation can be seen visibly by the user. |
Sharing of resource |
The sharing of resource-like procedures between different processes is difficult as page tables are required. |
The sharing of resources between different processes is easier as segments are facilitated to share. |
Use case |
Paging is used in general-purpose operating systems for efficient memory management. |
Segmentation is used in real-time OS, embedded systems, etc. |
Examples |
Paging is being used in Windows OS, MacOS, etc. |
Segmentation is used in the early versions of embedded systems, DOS, etc. |
Conclusion
Paging and Segmentation are both techniques of non-contiguous memory management in operating systems. In this article, we have learned about the differences between paging and segmentation, along with their characteristics, advantages, and disadvantages, and a simple program. We have seen in paging that the memory is divided into fixed-size pages, which results in internal fragmentation and page table overhead but also efficient memory consumption and streamlined allocation. Whereas, the memory is divided into variable-sized segments in the segmentation, which reduces internal fragmentation while increasing external fragmentation and complexity.
The decision between which technique to choose can be determined by the operating system’s specific requirements. By comprehending these memory management techniques, you can make informed decisions about optimising memory usage in your computer system.
FAQs
Paging can be slower than segmentation because of address translation overhead, memory access patterns, and the complexity of handling page faults.
Yes, both paging and segmentation can be used together to increase the performance of the system, and have the strengths of both techniques to manage the memory. The combination of both techniques is known as segmentation paging which is being used in modern operating systems.
Memory can be divided into variable-sized segments via segmentation, which corresponds to the logical structure of programs like functions, arrays, and
data structures. Because of this alignment, memory allocation is easier for programmers to manage and comprehend, and the representation is more logical.
No, both paging and segmentation are non-contiguous memory management techniques in operating systems.
By allocating memory to fixed-size pages and guaranteeing that each page is used to its maximum potential, paging removes internal fragmentation.