In Java, methods allow us to perform tasks in a structured way. Method overloading and method overriding are two major concepts in Java. To create multiple methods bearing the same name but different parameters, we may employ method overloading. Subclasses can provide a specific implementation for a method which has already been defined in its superclass through method overriding. This blog will explore what method overloading and method overriding refer to and how they differ from each other.
What is Method Overloading?
Method overloading in Java allows us to define multiple methods with the same name but different parameters. This makes our code more readable and organised since we can use the same method name for similar actions with different inputs. It is a way to achieve polymorphism in Java.
To achieve method overloading, you must change the method signature. The method signature includes the method name and the parameter list. You can change the number of parameters, the type of parameters, or both.
How to Achieve Method Overloading
Method overloading can be done by:
- Changing the number of parameters.
- Changing the type of parameters.
Example:
In the code below, we have three ‘add’ methods with different parameter lists. The first method adds two integers, the second adds three integers, and the third adds two doubles. When calling ‘example.add’, Java determines which method to invoke based on the arguments provided.
public class MethodOverloadingExample {
// Method with two int parameters
public int add(int a, int b) {
return a + b;
}
// Method with three int parameters
public int add(int a, int b, int c) {
return a + b + c;
}
// Method with two double parameters
public double add(double a, double b) {
return a + b;
}
public static void main(String[] args) {
MethodOverloadingExample example = new MethodOverloadingExample();
System.out.println(“Sum of 2 and 3: ” + example.add(2, 3)); // Calls the first method
System.out.println(“Sum of 2, 3, and 4: ” + example.add(2, 3, 4)); // Calls the second method
System.out.println(“Sum of 2.5 and 3.5: ” + example.add(2.5, 3.5)); // Calls the third method
}
}
Output:
Sum of 2 and 3: 5
Sum of 2, 3, and 4: 9
Sum of 2.5 and 3.5: 6.0
Advantages of Method Overloading
- Improved Readability: Making use of similar terms for related activities improves code simplicity and understandability.
- Ease of Use: Programs do not have to worry about different names for methods even though those methods call functions with different parameters.
- Code Maintenance: Organised management is possible when overloaded methods are grouped together logically.
- Consistency: This ensures that the code’s naming convention does not introduce any ambiguities or errors into the programming space.
- Flexibility: Methods can easily handle various types as well as numbers of inputs thereby making your codes more flexible.
- Cleaner Code: The fewer number of distinct operation names needed gives rise to a cleaner source code that is well arranged.
Also read: Inheritance in Java
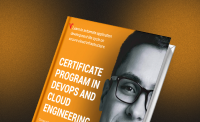
Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
What is Method Overriding?
Method overriding in Java allows a subclass to provide a specific implementation for a method that is already defined in its superclass. This feature enables polymorphism, where a subclass can modify or extend the behaviour of a parent class method.
How to Achieve Method Overriding
To achieve method overriding, the subclass must define a method with the same name, return type, and parameters as the method in its superclass. The @Override annotation is often used to indicate that a method is being overridden.
Example Code:
In the code below, the Animal class has a method called makeSound(). The Dog class extends Animal and overrides the makeSound() method to provide a specific implementation. When myAnimal.makeSound() is called, it uses the method from the Animal class. When myDog.makeSound() is called, it uses the overridden method in the Dog class, demonstrating polymorphism.
class Animal {
public void makeSound() {
System.out.println(“Animal makes a sound”);
}
}
class Dog extends Animal {
@Override
public void makeSound() {
System.out.println(“Dog barks”);
}
}
public class MethodOverridingExample {
public static void main(String[] args) {
Animal myAnimal = new Animal();
Animal myDog = new Dog();
myAnimal.makeSound(); // Calls the method from Animal class
myDog.makeSound(); // Calls the overridden method from Dog class
}
}
Output:
Animal makes a sound
Dog barks
Advantages of Method Overriding
- Runtime Polymorphism: An instance can change its behaviour dynamically at runtime by implementing this concept.
- Code Reusability: Reduces redundancy by allowing for the reuse of existing programs within one project.
- Improved Flexibility: When implemented by subclasses along with maintaining their parent classes’ interface, it allows them to define their own behaviours as well.
- Consistent Method Naming: Names assigned to different methods across classes will remain uniform throughout their lifespan.
- Simplifies Code: Avoiding conditional statements used in checking object types before calling the right methods.
- Enhanced Maintainability: Whenever there is a need to modify a method, one can do it in only one place (subclass) unlike doing it at multiple places.
- Extensibility: It helps introduce new features without the need to change code that has already been done on existing classes.
- Encapsulation: In addition, sub-classes may encapsulate respective code behaviours for the sake of organisation and readability.
Key Difference Between Method Overloading and Method Overriding in Java
Feature |
Method Overloading |
Method Overriding |
Definition |
Multiple methods with the same name but different parameters. |
Subclass provides a specific implementation of a superclass method. |
Parameters |
Different parameters (type, number, order). |
The same parameters are used for the method in the superclass. |
Return Type |
It can have different return types. |
It must have the same return type or a subtype. |
Scope |
Happens within the same class. |
Happens between superclass and subclass. |
Inheritance |
Not related to inheritance. |
Directly related to inheritance. |
Binding |
Compile-time binding (static). |
Runtime binding (dynamic). |
Annotation |
No annotation is required. |
Uses @Override annotation. |
Polymorphism |
Does not provide runtime polymorphism. |
Provides runtime polymorphism. |
Method Resolution |
Resolved at compile time. |
Resolved at runtime. |
Use Case |
Used to increase the readability of the program. |
Used to change the behaviour of the inherited method. |
Return Type Constraint |
No constraints on the return type. |
The return type must be the same or a subtype of the original. |
Exception Handling |
It can throw different exceptions. |
Can throw the same or fewer exceptions. |
Inheritance Requirement |
No inheritance is required. |
Requires inheritance (subclass-superclass relationship). |
Access Modifier |
Can have any access modifier. |
It cannot reduce the visibility of the inherited method. |
Impact on Performance |
Slightly affects compile time. |
It can affect runtime performance due to dynamic binding. |
Also read: Java Interview Questions
Conclusion
To become an effective Java programmer, it is essential to comprehend the differences between method overloading and method overriding. Method overloading enables us to apply the same name for a function but with different parameters, which makes our codes more readable and well-organised. On the other hand, method overriding allows a subclass to alter or extend the behaviour of an existing parent class’ method, thus promoting runtime polymorphism as well as code reuse.
You can write more flexible, maintainable and efficient code by mastering these concepts. Each technique has its own benefits and specific use cases; therefore, using them when necessary will help enhance programming skills. To learn Java development better, keep practising these concepts.
FAQs
Method overloading is defining multiple methods with the same name but different parameters.
Method overriding is when a subclass provides a specific implementation of a method already defined in its superclass.
Yes, method overloading can have different return types as long as the parameter lists are different.
While not required, the @Override annotation is recommended to indicate that a method is being overridden.
No, method overloading is not related to inheritance and occurs within the same class.
Yes, method overriding supports runtime polymorphism.
Yes, overloaded methods can throw different exceptions.