The variables are the building blocks of any program. It is used to store the data or values. It works as a container to store data. Through the variables, we interact with the different states of the code.
In this article, we will cover local and global variables and go through the differences between the two.
Variable in Python
A variable is a name that is associated with a value stored in some location. This value may be an elementary data type such as 2, 3.5, “abc”, or it can also be a complex data type like the list [2, 3, 4, 5] or dictionary {“a”: 1, “b”: 2}.
Unlike other languages Python does not require us to declare the variable explicitly or the types of the variables we use. Python implicitly handles this when we assign a value to the variable. The compiler implicitly understands the data type, and we can use the variable to store different types of data.
Examples:
a=2
b=”foo”
d=[1,2,3,4]
Scope in Python
The variables that are defined in the code have a different lifetime depending on the region it was called. The scope is defined as the region where a variable can be accessed.
In Python, there are three kinds of scopes:
- Local Scope
- Global Scope
- Non-Local Scope
Local Scope
The variables defined inside a function are only accessible inside the function. These variables have a local scope.
Global Scope
The variables defined outside any function or class have a global scope. These variables can be accessed from any part of the code.
Non-Local Scope
This type of scope is only applicable to the case of nested functions. In simple words, if a function is defined outside the inner nested function and rather in the outer function, then it is said to have a non-local scope.
Also Read: What are Python Functions
What Is a Local Variable?
In Python, a local variable could be any variable with a local scope, meaning it is defined inside a function and can only be accessed within the function. For instance:
Code:
def func():
a=2
print(a)
func()
Output:
2
In the above code, we defined a variable “a” inside the function “func()” and printed the value. In this code, the variable ‘a’ is the local variable, while the body of the function ‘func()’ is the local scope of the variable.
Now, you might wonder what will happen if we try to access ‘a’ outside of its scope. Let’s modify the code to check this for ourselves
Code:
def func():
a=2
func()
print(a)
Output:
NameError: name ‘a’ is not defined
In the above code, we have defined the variable ‘a’ inside the func() function. So, it has a local scope. If you try to access the variable ‘a’ outside of the function, it will throw an error.
Also Read: Data Structures and Algorithms in Python
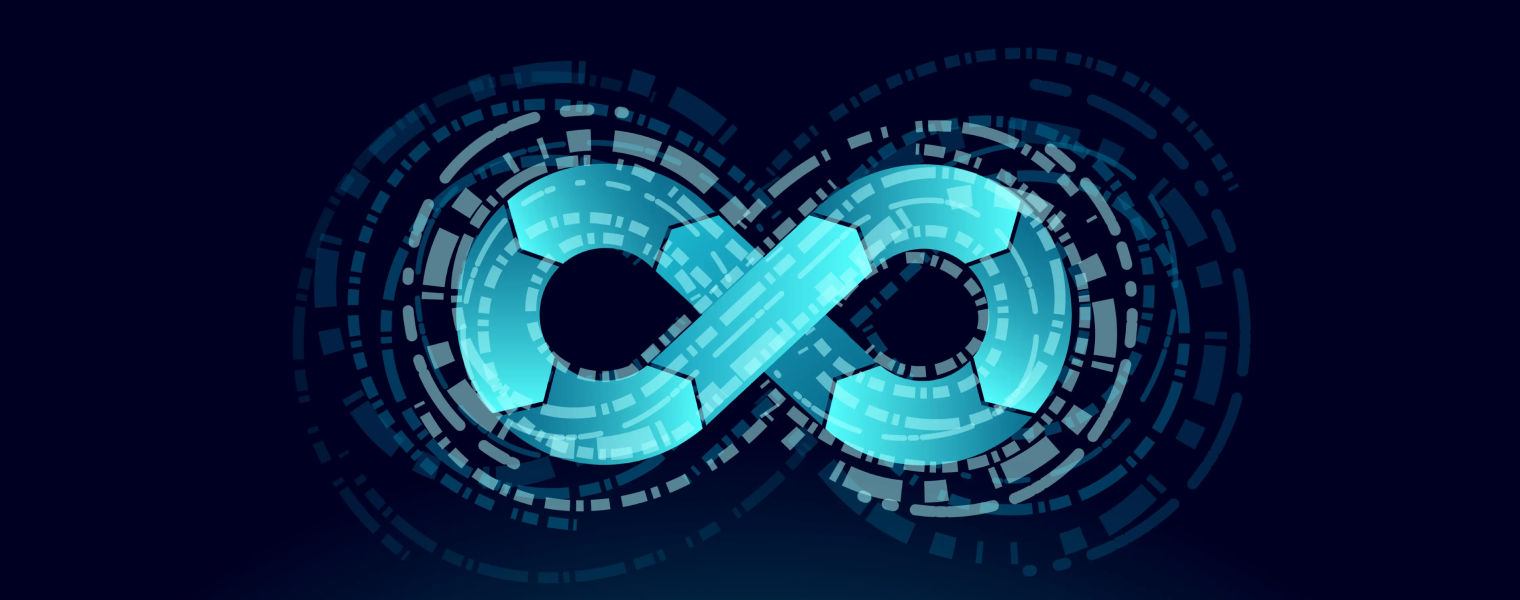
Internship Assurance
DevOps & Cloud Engineering
What is a Global Variable?
On the other hand, global variables are those that have been declared globally, meaning they can be accessed by any part of your program.
Let’s consider an example:
Code:
a=2
def func():
print(f”Inside func(): {a}”)
func()
print(f”Outside func(): {a}”)
Output:
Inside func(): 2
Outside func(): 2
In the above code, we defined a variable ‘a’ and a function ‘func()’. The variable ‘a’ is defined outside any function, so it is a global variable and as we can see from the output, we were able to access ‘a’ inside the function as well as outside the function.
What is a global Keyword?
The global keyword allows us to access a global variable in a local scope and modify it in ways that get reflected in the global scope.
Let’s go through an example to understand the necessity and use of global keywords:
Code:
a=2
def func():
a=3
print(f”Inside func(): {a}”)
func()
print(f”Outside func(): {a}”)
Output:
Inside func(): 3
Outside func(): 2
We modified the code used to understand global variables and added an assignment statement inside the function. By looking at the output, you might wonder what happened there. When we added the assignment statement, the compiler created a local variable ‘a’ inside the local scope of the function ‘func()’, which is separate from the global variable ‘a’ defined in the global scope. The print statement inside the function accessed the variable in the local scope, while the print statement defined outside the function accessed the variable in the global scope.
Now let’s look at another example, this time using the global keyword:
Code:
a=2
def func():
global a
a=3
print(f”Inside func(): {a}”)
func()
print(f”Outside func(): {a}”)
Output:
Inside func(): 3
Outside func(): 3
The only difference between this code and the previous code is the use of the global keyword. The global keyword inside the function ‘func()’ brings the global variable inside the local scope of the function. Hence, any assignments or modifications inside the function affect the global variable directly. Therefore, unlike the previous code, we see the same value for the variable ‘a’ irrespective of the scope in which the print statement was defined.
Difference Between Local and Global Variables in Python
Now, we have a clear understanding of local and global variables. Let’s understand the difference between local and global variables in Python.
|
Local Variables |
Global Variables |
Definition |
These variables are defined inside a function or class. |
These variables are defined outside any function or class |
Scope |
These variables have a local scope. |
These variables have a global scope. |
Accessibility |
These variables can only be accessed in the function it is defined in. |
These variables can be accessed from any part of the code. |
Lifetime |
These variables are created when the function is called and destroyed when the function execution stops. |
These variables are created when the program starts and remain in the memory throughout the duration of the program. |
Data Sharing |
These variables can’t be used for data sharing. |
These variables can be used for data sharing. |
Location |
These variables are stored in the fixed location selected by the compiler. |
These variables are stored in the stack. |
Also Read:
Conclusion
In this article, we learned the differences between local and global variables in Python. We began by examining what a variable is and its scope. From these ideas, we discussed the two types of variables: local and global variables. The concept of the global keyword was also well-explained as well as how it is used.
Finally, after understanding all the building blocks, we went through the differences between local and global variables in Python. We looked at it from a size point of view, definition, scope, accessibility, lifetime, data sharing and location.
FAQs
A variable is an identifier that represents a memory location where values can be stored.
Python has three types of scopes as follows: local scope, global scope, and nonlocal scope.
A local variable is any variable defined within a function or program block.
It refers to when you declare or create variables outside any function body in your program.
The global word inside code enables referring to variables defined inside other functions or even outside any functions.