Break and Continue statements are both part of C language that can manipulate the changes to the flow of the program, allowing for targeted execution. While both statements influence the flow, they serve distinct purposes. Break and Continue statements are also called jump statements, as by using these keywords, we can directly jump to the next part of a program which manipulates the flow of a program.
In this blog, we will dive into the functionalities of Break and Continue to see their key differences and provide practical code examples for a better understanding. Let’s learn more in detail about break and continue statements in c.
What is the Break Statement in C?
The break statement in C terminates the execution of a loop or switch statement entirely even having leftover conditions. When a break statement is encountered inside a loop or switch, the control is immediately transferred to the statement or causes the program to immediately jump out of following the loop or switch. The break statement is also known as an exit gate within a switch statement or a loop.
Syntax
break;
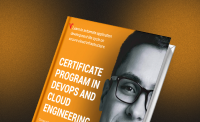
Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
How Does the Break Statement Work?
The break statement is used in loops like for, while, or a do-while loop and switch statements to exit from the current loop or switch structure.
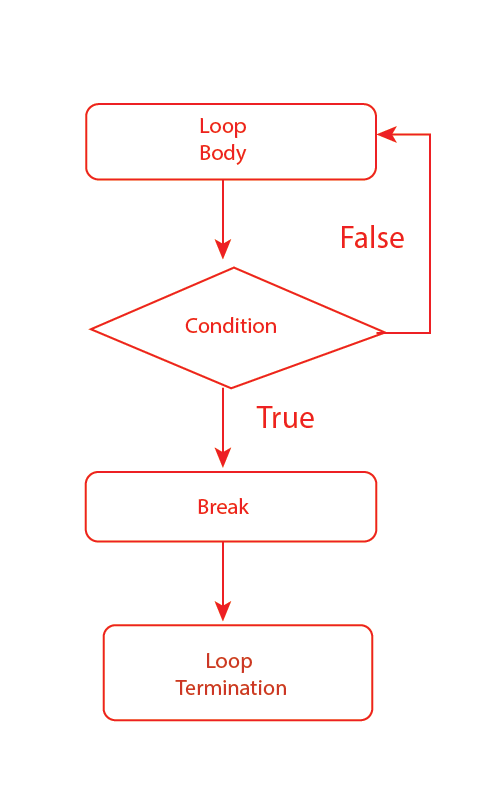
To see the complete working of a break statement, we have to see some code examples. See the following code examples to learn about how the break statement works:
Case 1: Inside a loop
The break statement can be used inside different loops available in C language, including a for, while, or a do-while loop. When used inside a loop for the execution, the control immediately exits the loop and proceeds with the next statement following the loop. See the following examples of using break statement in a C program:
1. Inside a For loop
Syntax:
for (init; condition; inc/dec) {
// other content
if (condition) {
break;
}
// More code content
}
Code Example: The below example demonstrates using a break statement inside a for loop in the C program.
#include <stdio.h>
int main() {
for (int i = 1; i <= 15; i++) {
if (i == 10) {
break; // Exit when i reaches 10 in the loop
}
printf(“%d “, i);
}
return 0;
}
Explanation:
In this example, we are using a break statement inside a for loop. Here, the loop iterates from i = 1 and runs as long as i <= 15, but when i reaches 10, the if condition triggers, and the break statement terminates the loop immediately.
Output:
1 2 3 4 5 6 7 8 9
2. Inside a While loop
Syntax:
while (condition) {
// other content
if (conditionTwo) {
break;
}
// More code content
}
Code Example: The below example demonstrates using a break statement inside a while loop in a C program.
#include <stdio.h>
int main() {
int i = 1;
while (i <= 15) {
if (i == 10) {
break; // Exit the while loop when i reaches 10
}
printf(“%d “, i);
i++;
}
return 0;
}
Explanation:
In this example, we use a break statement inside a while loop. Here, the loop iterates from 1 to 10 and prints all the numbers (i) before exiting. But here the condition (i <= 15) is still true when i reaches 10, and finally, the break statement forces the loop to exit or terminate.
Output:
1 2 3 4 5 6 7 8 9
- Inside a do-while loop
Syntax:
do {
// other content
if (conditionTwo) {
break;
}
// More code content
} while (condition);
Code Example: The below example demonstrates using a break statement inside a do-while loop in a C program.
#include <stdio.h>
int main() {
int i = 1;
do {
if (i == 10) {
break; // Exit the while loop when i reach 10
}
printf(“%d “, i);
i++;
} while (i < 10);
return 0;
}
Explanation:
In this example, we are using a break statement inside a do-while loop. Here, the loop iterates i from 1 to 10 and prints all the numbers i.e., i, before terminating with the break statement.
Output:
1 2 3 4 5 6 7 8 9
Case 2: Inside a Switch
The break statement can also be used inside a switch statement in a C program. When executed within a switch case, the break statement forces the code to exit the switch structure immediately and continue with the next statement written after the switch.
Syntax:
switch (myNum) {
case 1:
printf(“printing case 1n”);
break;
case 2:
…
}
Code Example:
#include <stdio.h>
int main() {
int num = 5;
switch (num) {
case 1:
printf(“Rendering Case 1n”);
break;
case 2:
printf(“Rendering Case 2n”);
break;
case 3:
printf(“Rendering Case 3n”);
break;
case 4:
printf(“Rendering Case 4n”);
break;
case 5:
printf(“Rendering Case 5n”);
break;
case 6:
printf(“Rendering Case 6n”);
break;
case 7:
printf(“Rendering Case 7n”);
break;
case 8:
printf(“Rendering Case 8n”);
break;
case 9:
printf(“Rendering Case 9n”);
break;
default:
printf(“Rendering Case 10 which is defaultn”);
}
return 0;
}
Explanation:
In the example, When num is 5, the case 5 block executes and prints “Rendering Case 5”. But if we don’t use the break statement, execution “falls through” to subsequent cases, printing all the cases after 5.
Output:
Rendering Case 5
Also read: Difference Between While and Do While Loop in C
What is the Continue Statement in C?
The continue statement in C allows us to skip the remaining code inside the loop for the current iteration only, after which the subsequent iteration of the loop is then carried out. Unlike break, it doesn’t terminate the loop entirely. But it re-checks the condition and continues as usual as a fresh program loop runs.
In easier words, the continue statement allows moving to the next iteration while not terminating the entire execution or exiting from the program but only the current loop iteration. Note that a continue statement will only be used with the loops, including for, while, or do-while, and not with the switch case (as in the break statement).
Syntax
continue;
How Does the Continue Statement Work?
The working of the continue statement is quite easy. As told above, the continue statement doesn’t work with the switch but only with the loops.
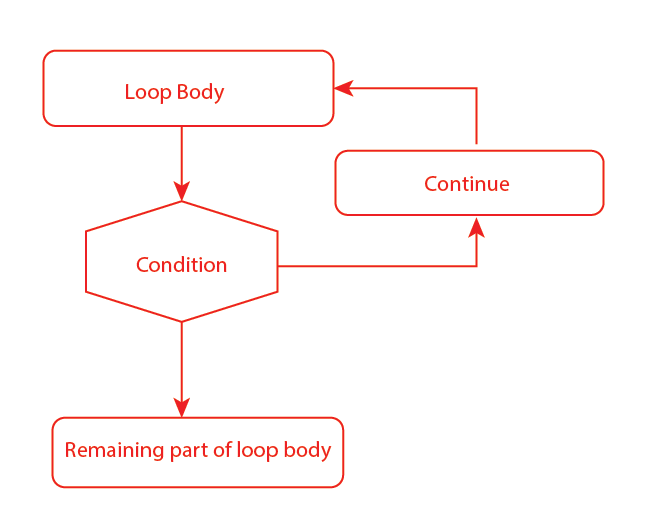
We use the continue statement within loops to skip the current iteration and directly jump to the next iteration.
Case 1: Using inside a for loop
The break statement can be used inside a loop like a for loop. When the continue statement is executed, the control skips the remaining code inside the loop for the current iteration and immediately jumps to the beginning of the next iteration.
Syntax:
for (init; condition; inc/dec) {
// other content
if (condition) {
continue;
}
// More code content
}
Code Example: The below example demonstrates the usage of the continue statement inside a for loop.
#include <stdio.h>
int main() {
for (int i = 0; i <= 15; i++) {
if (i % 3 == 0) {
continue; // continue when the i%3 remainder gets 0
}
printf(“%d “, i);
}
}
Explanation:
In this example, we use a continue statement inside a for loop. Here, the loop iterates from 0 to 15. When an if statement is triggered, it skips all multiples of 3 (0, 3, 6, 9, 12) using continue, and finally, the printf statement then prints the remaining non-multiples of 3.
Output:
1 2 4 5 7 8 10 11 13 14
Case 2: Using inside a while loop
Syntax:
while (condition) {
// other content
if (conditionTwo) {
continue;
}
// More code content
}
Code Example: The below example demonstrates the usage of the continue statement inside a while loop.
#include <stdio.h>
int main() {
int i = 1;
while (i <= 20) {
if (i % 2 == 0) {
i++;
continue; //continues or skips when remainder of i % 3 gets 0
}
printf(“%d “, i);
i++;
}
}
Explanation:
In this example, we use a continue statement inside a while loop. The loop iterates from 1 to 20. When the if statement is triggered, it skips all even numbers (2, 4, 6, 8, 10, 12, 14, 16, 18, and 20) using continue and finally prints all the remaining odd numbers.
Output:
1 3 5 7 9 11 13 15 17 19
Case 3: Using inside a do-while loop
Syntax:
do {
// other content
if (conditionTwo) {
continue;
}
// More code content
} while (condition);
Code Example: The below example demonstrates the usage of the continue statement inside a do-while loop.
#include <stdio.h>
int main() {
int i = 1;
do {
if (i % 3 == 0) {
i++;
continue; //continues or skips when remainder of i % 3 gets 0
}
printf(“%d “, i);
i++;
} while (i <= 20);
return 0;
}
Explanation:
In this example, we are using a continue statement inside a do-while loop. The loop iterates from 1 to 20. When the if statement is triggered, it skips all multiples of 3 (3, 6, 9, 12, 15, and 18) using continue and then prints the remaining nondivisible numbers of 3.
Output:
1 3 5 7 9 11 13 15 17 19
Difference Between Break and Continue Statement in C
Some of the key differences between the break and continue statements are given below:
Parameters |
Break Statement |
Continue Statement |
Use Case |
The break statement can be used to terminate/exit the loop or a switch case. |
The continue statement can be used to skip or jump to the next iteration of a loop. |
Context |
The context of the break statement is in loops and switch cases. |
The context of usage is only in loops. |
Effect on Loop |
It ends the loop or switch iteration. |
It continues to the next iteration. |
Effect on Switch |
Using break exits the switch statement. |
The switch statement is not applicable in continue. |
Placement in Code |
It can be used in any loop including for, while, or do-while loop and switch. |
It can be placed in loops only. |
Exit point |
It exits completely from the loop. |
It jumps to the next iteration only. |
Complexity |
Complexity is simpler and easy to use if not used in nested loops. |
It is easier and can make the code look cleaner by skipping the unnecessary conditions. |
Scope |
It affects the scope used within. |
It affects the current iteration of the loop. |
Code Execution |
The code executed is stopped immediately. |
Skips the remaining code in the current execution. |
Error Handling |
Error handling is a common use case of break. |
Error handling is used here to skip the unnecessary conditions to avoid future issues. |
Loop counter |
It may terminate before the loop counter is used up. |
Here, the loop counter is normal. |
Syntax |
break; |
continue; |
Conclusion
In this blog, we learned the differences between the break and continue statements in C. Understanding the concepts of both these statements helps you determine where to use a break statement and where to use the continue statement for an effective loop control in your C program. While both offer you to alter the loop flow control the use cases we learned in this blog differ.
The break statement is used to exit the loop or switch entirely, whereas the continue statement is used to skip or jump to the next iteration of the loop. Learning the techniques of using break and continue statements can help you effectively manage the loop structure in the C program.
FAQs
In a do-while loop, the condition is checked after the loop body executes at least once. Therefore, even if you place continue before the condition, the first iteration will always be executed regardless.
Yes, we can use both break and continue statements simultaneously in a C program.
The key difference between both is that a break statement is used to terminate the loop entirely while continue is used to skip/jump to the next iteration.
The break statement is used in for, while, and do-while loops and also in the switch statement, while the continue statement is used only in the for,
while, and do-while loops.
The break is a type of statement used in C language that brings the program control out of the loop, or in simpler terms, it basically terminates the loop entirely.