Given the complexity of software engineering, Abstraction is a critical element to consider when programming in Python. Abstraction helps developers simplify processes by grouping related tasks and ignoring the details that are not essential to the project. By abstracting away unnecessary complexities, Python developers can better focus on more important aspects of their projects, such as efficiency or scalability. The ability to abstract data and classes in Python allows developers to create code that’s easier to maintain and understand. In this article, we’ll take a closer look at abstraction in Python, discussing what it is, how it works, and examples of how it’s used.
Explore Business Analytics and Data Science Course
What is Abstraction in Python?
The term “abstraction” in Python refers to the process of taking complex concepts and simplifying them. In other words, abstraction is the practice of hiding away information that isn’t necessary or relevant to a particular task. For example, if you were writing code to calculate the price of an item, you wouldn’t need to write out every line of code; instead, you could rely on an abstracted version like “item_price = item_cost + tax”.
In Python, abstraction can be achieved through data abstraction and class abstraction. Data abstraction hides implementation details and makes it easier for developers to understand how their programs are structured. This includes using functions or classes as parameters so that programmers don’t have to worry about the implementation details. On the other hand, class abstraction is used for creating abstract base classes that provide a template for creating subclasses and defining their methods.
What Is a List in Python?
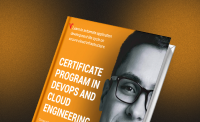
Get curriculum highlights, career paths, industry insights and accelerate your technology journey.
Download brochure
How Abstraction Simplifies Complex Concepts?
Abstraction allows developers to simplify complex concepts, making it easier for programmers to understand the code structure and focus on more important tasks. When it comes to coding in Python, abstraction can be a powerful tool for managing complexity. By abstracting away unnecessary details, coders can create shorter, more efficient programs with fewer lines of code that are easier to debug.
One of the key advantages of abstraction is that it helps reduce redundancy in code by preventing developers from having to write out the same line of code multiple times. Abstraction also makes software easier to maintain as changes only need to be made in one place instead of several different locations within the program. Furthermore, abstraction helps improve scalability by allowing programs to be quickly adapted to changing requirements or environments.
Benefits and Importance of Abstraction in Python
- Makes code easier to understand and maintain
- Reduces redundancy in code
- Improves scalability
- Allows developers to focus on important aspects of programming, such as efficiency or scalability
- Helps developers create shorter, more efficient programs with fewer lines of code that are easier to debug.
- Makes software easier to maintain by allowing changes only to be made in one place instead of multiple locations within the program.
Learn About Tuple in Python
Abstraction Techniques in Python
Python offers several techniques for abstracting away unnecessary details. These include:
- Using functions or classes as parameters
- Creating abstract class python
- Leveraging templates to streamline processes
- Organizing data into distinct categories
- Hiding implementation details with data abstraction
Abstraction in Python works by hiding complex processes and details that may not be relevant to the task at hand. This involves using functions or classes as parameters so that programmers don’t have to worry about the implementation details. One of the most common ways abstraction is used in Python is through abstract base classes (ABCs). An ABC allows developers to create a template for creating subclasses and defining their methods. By leveraging these templates, Python developers can streamline processes and avoid writing out each line of code multiple times.
How do These Techniques Help in Creating Abstract and Reusable Code?
Using functions or classes as parameters allows for more concise code that is easier to maintain and debug. Additionally, creating abstract base classes (ABCs) provides a template for creating subclasses and defining their methods, making the code more organized and structured. Furthermore, organizing data into distinct categories helps keep the project’s structure intuitive while hiding implementation details with data abstraction prevents users from having to worry about unnecessary complexities.
Creating Abstract Classes and Interfaces in Python
Abstract classes and interfaces are two of the most common ways abstraction is used in Python. An abstract class is a template for creating subclasses that define their methods without providing implementation details. On the other hand, an interface allows developers to define what functionality must be provided by each subclass while leaving it up to the subclass to provide its own implementation details.
Why use Abstract Base Class?
Using an abstract base class is useful for creating reusable code. It allows developers to define a template that all subclasses must follow while leaving it up to the subclass to provide its own implementation details. This helps keep code organized and structured, making it easier to maintain. Additionally, using an ABC can help reduce redundancy in code by preventing developers from having to write out each line of code multiple times.
An example of using an abstract base class is when creating classes for different types of shapes. By leveraging an ABC, developers can easily create new shapes (like circles or squares) with their own methods and attributes without having to define them each time a shape is created.
What is NumPy in Python?
Methods in Abstract Base Class
Methods in an abstract base class allow developers to define what functionality is shared by all subclasses. By leveraging methods, coders can easily create new classes while ensuring that they all share common attributes and behaviors. This helps keep code organized and makes it easier to maintain as changes only need to be made in one place instead of several different locations within the program.
An example of using a method in an ABC would be when creating a shape class for two-dimensional shapes like circles or squares. The ABC would provide each subclass with a set of methods (such as getArea() or draw()) that will be inherited by all subclasses without having to redefine them each time a subclass is created.
Abstract Class Instantiation
It is impossible to instantiate an abstract class in Python. While it is possible to define an abstract class, the main purpose of an ABC is to provide a template for creating subclasses that can be instantiated and used. In order to create an instance of a subclass, developers must first define their own implementation details before they can create any objects from the subclass. When you create a subclass of an abstract class, the subclass will provide implementations for all the abstract methods that were defined in the parent class.
Real-world Examples of Abstraction in Python
- Graphical User Interfaces (GUIs): Abstraction in Python is used to create graphical user interfaces (GUIs) that hide away code complexity from users while allowing them to interact with the program in a more intuitive way.
- Database Access: Database access also makes use of abstraction in Python by hiding away details such as query syntax so developers can focus on creating efficient data processing pipelines without worrying about underlying implementation details.
- Machine Learning and AI Applications: Abstraction techniques like templates are often used for machine learning and artificial intelligence applications, where the goal is to write code that is short, concise, and efficient while still providing accurate results.
- Networking Applications: Abstraction in Python is also used for building networking applications. By leveraging code abstraction techniques, developers can easily create complex client-server networks without having to worry about the underlying implementation details.
- APIs: Abstraction in Python is also used for creating Application Programming Interfaces (APIs) that allow different programs to communicate without worrying about the underlying implementation details.
Best Practices for Abstraction in Python
- Use abstract classes and interfaces to create templates for creating subclasses and defining their methods.
- Leverage functions or classes as parameters to keep code concise and organized.
- Employ data abstraction techniques to hide away implementation details from users so they can better focus on understanding the structure of the program.
- Take advantage of inheritance when it comes to writing more efficient code with fewer bugs by avoiding unnecessary redundancies in code, especially when dealing with complex programs.
- Ensure all methods are properly defined in the parent class before instantiating any objects from a subclass.
- Test your code often, especially after making changes, to ensure that everything is working properly as intended and there are no errors.
- Remember to document your code where necessary, as this will help you and other developers better understand the structure of the program.
- Try not to overuse abstraction when it comes to writing code, as this may lead to confusing and inefficient code that is difficult to maintain.
Conclusion
Abstraction is an incredibly important concept that makes it easy for developers to create complex programs without having to worry about underlying implementation details. By utilizing abstract classes and interfaces, developers can easily create templates for creating subclasses while avoiding unnecessary redundancies in code.
FAQs
Some best practices for effective abstraction in Python include using abstract classes and interfaces to create templates for creating subclasses, leveraging functions or classes as parameters to keep code concise and organized, employing data abstraction techniques to hide away implementation details from users, and taking advantage of inheritance when it comes to writing efficient code with fewer bugs.
Encapsulation is an important concept when it comes to abstraction in Python, as it allows developers to hide away implementation details from users while still providing them with the necessary functionality. This helps ensure that code is organized and maintainable while also reducing the amount of redundant code written.
Some common abstraction techniques used in Python include abstract classes, interfaces, functions/classes as parameters, data abstraction methods, and inheritance. These techniques are used to create templates for creating subclasses while avoiding unnecessary redundancies in code. They also help ensure that code is organized and maintainable while still providing users with the necessary functionality.
Inheritance helps promote abstraction and code reusability in Python by allowing developers to define methods in the parent class that can be inherited by all subclasses without having to redefine them each time a subclass is created. This eliminates unnecessary redundancies in code while still providing users with the necessary functionality. It also ensures that code is organized and maintainable, making it easier for developers to understand and maintain complex programs.